To provide executives with access to business data on the go, enterprises are adopting iPhones and iPads in droves. Many of these applications need innovative data visualizations but are currently restricted by the limited capabilities of available charting libraries for iOS. Some have limited chart types, whereas others lack visual appeal and interactivity. Open-source libraries are not backed by proper technical support either. So what are your options?
Enter FusionCharts XT, which addresses all these concerns. Being a JavaScript charting library, it can quickly render charts and widgets within the UIWebView control of an iOS application. With over 90 chart types that are animated and interactive, it augments the visual appeal and functionality of your iOS applications. In addition, dedicated tech support is there to answer any or all of your queries.
You can either obtain the data from a remote web service or access the local data stores on the device. This data needs to be provided in XML or JSON format that the FusionCharts JavaScript graph object understands. This blog post will show you how to use FusionCharts XT in an iOS app and power it using data from a web service. We will create the necessary XML and HTML required for the UIWebView to display our chart.
We now need to add FusionCharts XT’s JavaScript files to our project. Copy the JavaScript files present in
Add the JSONKit files, namely
We now need to connect the UIWebView outlet to this UIWebView. From the Document Window in Interface Builder, Ctrl+Drag from
After this, let us concern ourselves with the HTTP query and handling its JSON response.
Uncomment the
Table of Contents
Example – An iOS App to Plot Twitter Data Using FusionCharts XT
We will be plotting the count of mentions of ‘HTML5’ for each of the last seven days in a UIWebView control using FusionCharts XT. This is how your chart will finally look like in an iPhone: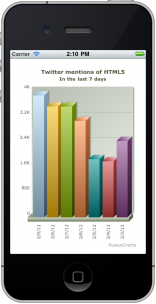
Requirements
- Xcode 3.2 and above
- The latest version of FusionCharts XT
- JSONKit library
- You would need to have internet access too. We will be using the
searchhistogram
query from the Topsy API. The exact query ishttp://otter.topsy.com/searchhistogram.json?q=html5&slice=86400&period=7
. You may run this query and see the JSON response, which returns the number of times ‘HTML5’ was mentioned every day for the last seven days.
How We Will Create the Chart
To create the chart, we will go through the following steps:- Create a View-based project in Xcode
- Use Topsy’s Otter API to get the count of mentions of ‘HTML5’ over the last seven days
- Use JSONKit to parse through the received JSON response
- Create the XML data for the chart
- Create the HTML required to show the chart
- Enable rotation of the chart according to the device’s orientation so that the chart will fit the screen whether the device is in landscape or portrait mode
Creating the Xcode Project
Let us open Xcode and create a View-based project, and save it as FusionChartsXTiOSDemo.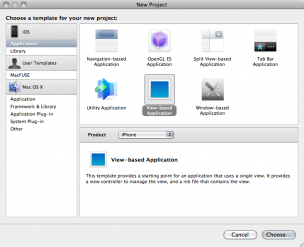
Download Package > Charts
to the Xcode Resources project group. Make sure you select the Copy items into destination group’s folder (if needed)
checkbox on the top. Doing so would ensure that you have a copy of all the JavaScript files local to your application. Click Add
to add your files to your project.
We need to modify Xcode’s compilation step a little bit. Xcode considers JavaScript files as code (rightfully so) and tries to compile them. However, we want these files to render charts inside our UIWebView and not compiled. Expand the Targets
group and your project target. Our target is called FusionChartsXTiOSDemo
. Also, expand the Copy Bundle Resources
and Compile Sources
build phases. Next, select the JavaScript files and drag them from the compile stage to the copy bundle build phase.
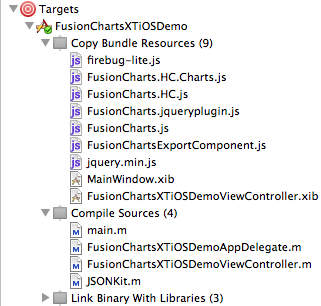
JSONKit.h
and JSONKit.m
to your project too. We have now prepared Xcode with all the files necessary. Let us begin designing and coding!
Designing and Coding
InFusionChartsXTiOSDemo.h
, add an IBOutlet
for the WebView.
@property (nonatomic, retain) IBOutlet UIWebView *webView;
Also, @synthesize
and release
this accordingly.
Open your project’s main view controller Interface Builder file (inside the Resources group). For our project this file is called FusionChartsXTiOSDemoViewController.xib
. To add a WebView control to your app, drag a UIWebView from Interface Builder’s library to our view.
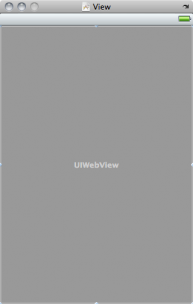
File’s Owner
to the UIWebView
. You should get a popup bubble, click webView
. Save your work and close Interface Builder.
Next, we will add the required properties needed to create the chart data and its configuration. We will write the following code in FusionChartsXTiOSDemo.h
:
// Chart properties. @property (nonatomic, retain) NSMutableString *htmlContent; @property (nonatomic, retain) NSMutableString *javascriptPath; @property (nonatomic, retain) NSMutableString *chartData; @property (nonatomic, retain) NSMutableString *chartType; @property (nonatomic, assign) UIInterfaceOrientation currentOrientation; @property (nonatomic, assign) CGFloat chartWidth; @property (nonatomic, assign) CGFloat chartHeight; @property (nonatomic, retain) NSMutableString *debugMode; @property (nonatomic, retain) NSMutableString *registerWithJavaScript;Let us also add the properties we require to perform the HTTP request and to handle the response data:
// Twitter data. @property (nonatomic, retain) NSMutableString *twitterQuery; @property (nonatomic, retain) NSMutableData *twitterData; @property (nonatomic, retain) NSDictionary *twitterDataDictionary; @property (nonatomic, assign) BOOL twitterDataError;Remember to
@synthesize
and release
the above properties correctly.
Declare the following 4 methods here; we will define them later.
- (void)displayDataError; - (void)createChartData:(UIInterfaceOrientation)interfaceOrientation; - (void)plotChart; - (void)removeChart;As a best practice, let us keep our first task to handle any error that might crop up because of connectivity issues. For this, we need to define the
displayDataError
method. We need to create the HTML that displays the error in plain English to the user in his WebView.
- (void)displayDataError { NSMutableString *displayErrorHTML = [NSMutableString stringWithString:@""]; [displayErrorHTML appendString:@""]; [displayErrorHTML appendString:@""]; [displayErrorHTML appendString:@"Unable to plot chart. Error receiving data from Twitter. "]; [displayErrorHTML appendString:@""]; [self.webView loadHTMLString:displayErrorHTML baseURL:nil]; }We have created the HTML content to show in the WebView in case of a network error or in case the Topsy API is unable to respond. Also, we could simulate a network error for testing this method either by unplugging the LAN cable or turning off the WiFi. When you’ve done either of these, run the project, and this is the error that you should see:
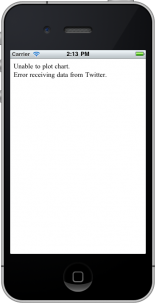
viewDidLoad
method, and create and execute the query to Topsy’s API:
// Setting up the Twitter query. self.twitterQuery = [NSMutableString stringWithFormat:@"%@", @"http://otter.topsy.com/searchhistogram.json?q=html5&slice=86400&period=7"]; NSURLRequest *request = [NSURLRequest requestWithURL:[NSURL URLWithString:self.twitterQuery]]; NSURLConnection *connection = [[NSURLConnection alloc] initWithRequest:request delegate:self]; // Check whether we have a valid connection. if (connection) { // Create the NSMutableData to hold the received data. self.twitterData = [NSMutableData data]; } else { // Error in receiving data. self.twitterDataError = YES; } // Done using the connection. [connection release];Now that we have made the connection, we need to prepare the four delegates of
NSURLConnection
.
We should reset all previous data each time we receive a message at connection:didReceiveResponse
.
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response { // This method is called when the server has determined that it // has enough information to create the NSURLResponse. // It can be called multiple times, for example, in the case of a // redirect, so each time we reset the data. // (Re)Initialize the Twitter data store. [self.twitterData setLength:0]; }Let us implement the delegate that stores the newly sent data.
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data { // Store received data. [self.twitterData appendData:data]; }If NSURLConnection returns an error while loading the data, let us explain it in plain English to the user using our own method
displayDataError
.
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error { // Display an error on connection failure. [self displayDataError]; }Finally, using JSONKit, let us convert the data stored in
self.twitterData
to a dictionary. Once the data has been converted, we call the createChartData::(UIInterfaceOrientation)interfaceOrientation
method.
- (void)connectionDidFinishLoading:(NSURLConnection *)connection { // Convert received JSON data to an Objective-C dictionary. self.twitterDataDictionary = [self.twitterData objectFromJSONData]; // Create chart as per current orientation. self.currentOrientation = self.interfaceOrientation; [self createChartData:self.currentOrientation]; }In the
createChartData::(UIInterfaceOrientation)interfaceOrientation
method, we need to parse through the dictionary and form our XML. Note that the dictionary has two keys named request
and response
. Within response
, there is an array named histogram
, which holds the count of mentions of ‘HTML5’ for the last seven days. We need to get hold of this array in NSArray
.
- (void)createChartData:(UIInterfaceOrientation)interfaceOrientation { // Check whether we have valid data. if (self.twitterDataError) { [self displayDataError]; } else { // Valid data. self.chartWidth = 300; self.chartHeight = 440; // Setup chart XML. NSDictionary *responseData = [self.twitterDataDictionary objectForKey:@"response"]; NSArray *histogramData = [responseData objectForKey:@"histogram"];We continue the same code block to iterate through
histogramData
and form the chart data in XML. Since the numbers in the array are for the previous seven days, starting from yesterday, we need to calculate and format the date accordingly.
self.chartData = [NSMutableString string]; [self.chartData appendFormat:@""]; NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init]; [dateFormatter setDateStyle:NSDateFormatterShortStyle]; for (int i = 0; i < [histogramData count]; i++) { [self.chartData appendFormat:@"", [dateFormatter stringFromDate:[NSDate dateWithTimeIntervalSinceNow:-(i+1)*86400]], [histogramData objectAtIndex:i]]; } [self.chartData appendFormat:@""]; [dateFormatter release];In the same code block, we create the HTML required to show the chart. Note the path of
FusionCharts.js
in the
tag. We will provide the base URL when we actually load the HTML in the WebView.
// Setup chart HTML. self.htmlContent = [NSMutableString stringWithFormat:@"%@", @""]; [self.htmlContent appendString:@""]; [self.htmlContent appendString:@"
Chart will render here.
"]; [self.htmlContent appendString:@""];Finally, we send the
plotChart
message to self
.
// Draw the actual chart. [self plotChart]; } }Thereafter, we will define the
plotChart
method. In this method we will provide the baseURL
, and load the HTML string we created.
- (void)plotChart { NSURL *baseURL = [NSURL fileURLWithPath:[NSString stringWithFormat:@"%@", [[NSBundle mainBundle] bundlePath]]]; [self.webView loadHTMLString:self.htmlContent baseURL:baseURL]; }Run this project in the iPhone Simulator, you would get the following chart:
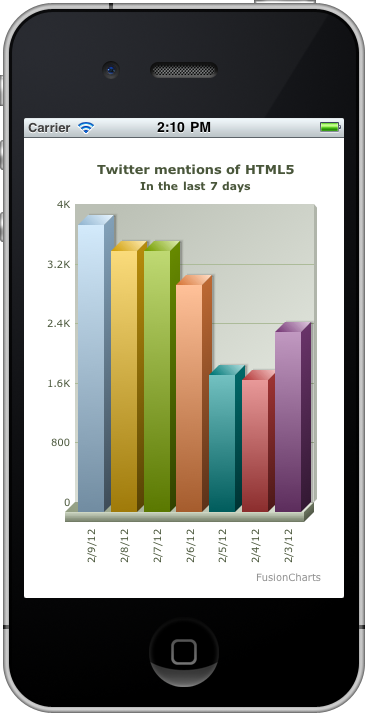
Making FusionCharts Aware of the Orientation
However, our work here is not done yet. We still need to add orientation support to this project. Let us override the default value to allow autorotation according to the orientation.// Override to allow orientations other than the default portrait orientation. - (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation { // Return YES since we support all orientations. return YES; }We need to dispose of the chart before we render it again according to the new orientation. To do this, we can can empty
chart_container
of all the HTML. Let us write the removeChart
method to do this.
- (void)removeChart { NSString *emptyChartContainer = [NSString stringWithString:@""]; [self.webView stringByEvaluatingJavaScriptFromString:emptyChartContainer]; }Next, we need to know exactly when the device begins to rotate. For this, we have the
)willRotateToInterfaceOrientation:(UIInterfaceOrientation)toInterfaceOrientation duration:(NSTimeInterval)duration
class method. In this method, we will first check if the data is valid; so that an error message will be displayed even upon rotation. Next, we store the orientation to which the device is rotating to. Then we remove the chart and render it again according to the new orientation.
// Handle interface rotation. - (void)willRotateToInterfaceOrientation:(UIInterfaceOrientation)toInterfaceOrientation duration:(NSTimeInterval)duration { // Check whether we have valid data. if (self.twitterDataError) { [self displayDataError]; } else { // Valid data. // Store new orientation. self.currentOrientation = toInterfaceOrientation; // Remove existing chart and recreating it // as per the new orientation. [self removeChart]; [self createChartData:self.currentOrientation]; } }In the
createChart
method, we need to supply the new chart dimensions to the FusionCharts object according to the orientation. So let us add the following code just before the block where we create the chart XML:
// Set chart width and height depending on the screen's orientation. if (interfaceOrientation == UIInterfaceOrientationPortrait || interfaceOrientation == UIInterfaceOrientationPortraitUpsideDown) { self.chartWidth = 300; self.chartHeight = 440; } else if (interfaceOrientation == UIInterfaceOrientationLandscapeLeft || interfaceOrientation == UIInterfaceOrientationLandscapeRight) { self.chartWidth = 440; self.chartHeight = 280; }Let us now run this project. Rotate your iPhone Simulator by ⌘+Left Arrow or ⌘+Right Arrow.
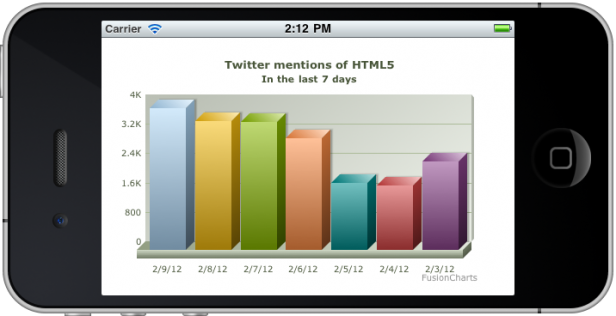
Download Sample Project
The sample project that we created is available for download. We used Xcode 3.2 and the target was iOS 4.2.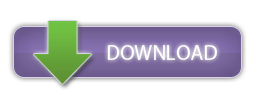
Rowena Sanchez
March 29, 2012, 11:12 amDo you have one for Android?
Hrishikesh Choudhari
March 29, 2012, 11:24 amHi Rowena,
You will have to use the WebView component provided in the Android SDK.
In the WebView, you can create charts using a similar methodology illustrated in this post.
Let us know when you try it in Android..
Saikat
April 4, 2012, 2:45 pm[self.htmlContent appendFormat:@”var chart_object = new FusionCharts(‘Column3D.swf’, ‘twitter_data_chart’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
What is the significance of ‘0’, ‘1’ in the HTML code?
Hrishikesh Choudhari
April 4, 2012, 2:57 pmHi Saikat,
The FusionCharts constructor takes in parameters as follows:
– Chart’s SWF name: This is automatically mapped to the JavaScript alias when rendering in JavaScript mode.
– Unique ID for chart: All charts should have unique IDs
– Width and Height: Dimensions can be specified in pixels or in percentages. When specified in percentages, the chart will relate to the container element.
– Enable Debug Mode: This is a Boolean which controls the Debug Window when rendered in Flash. For debugging in JavaScript, please refer to the JavaScript Debugging Documentation.
– Register with JavaScript: This parameter allow the FusionCharts JavaScript Object to interact with the chart. This is always set to ‘1’ internally.
Juan Gonzalez
April 11, 2012, 7:36 pmHi, great tutorial. This is the one that finally helped me run FC inside an iOS app. Great work!
Now that I have the example working I’d like to go a bit further and refactor the code a bit. I’d like to run it by you if it’s ok to be sure I’m understanding it all.
Besides connection checks and methods, the main events happen in createChartData:interfaceOrientation. As I see it this method does the following:
1- Check for valid data
2- Setup chart size
3- Setup chart XML and HTML
4- Draw chart
I want to refactor this code using MVC and I believe the separation should something like this:
(1) and (2) belong to the controller
(3) belongs to the model
(4) belongs to the view
Is that correct or am I missing something?
I’d like to be able to put everything in its place because when I start adding different data sources and reports this will get messy.
Thanks in advanced for this great post and for your help.
Best regards,
Hrishikesh Choudhari
April 12, 2012, 1:24 pmHi Juan,
We’re glad that you liked our tutorial.
The points you illustrate about refactoring the code to follow MVC are correct.
We believe you can further refine your code in this way:
Model:
(1) Retrieve the data
(2) Check for its validity
Controller:
(1) Setup chart size
(2) Setup the required XML and HTML
(3) Call the View from the Controller to draw the chart
View:
(1) Display the chart
Essentially we’re are calling the View from the Controller itself. The code required to draw the chart should be in the Controller, while the View should simply interpret this code and render the chart.
Let us know if this works for you..
Juan Gonzalez
April 12, 2012, 3:59 pmHi, thanks!
I’m trying to make it work but I’m having troubles passing some info between classes.
Do you have by any chance an example (code) of some MVC scenario that I can look at and extrapolate to this one? Maybe some other tutorial that I can check and figure it out?
I’ll keep on trying. Thanks again for the help.
Regards,
ragav
July 6, 2012, 10:49 amHi,
Latest version fusionchart support in android 4.0 .Is it possible lower version android.Is there any solution?
Hrishikesh Choudhari
July 6, 2012, 11:07 amHi Ragav,
FusionCharts XT should work in all versions of the Android OS.
Are you facing any issues? Please let us know at [email protected] and we’ll help you out.
ragav
July 19, 2012, 8:50 amHi Hrishikesh,
1.Yes, FusionCharts XT should work in above 3.0+ for me in android.Then how to figure out chart orientation in various android mobile screen?
2.Then can i write click event on fusion charts.Right now i can write click event on HTML div.But my requierment is click event on charts.
ragav
July 19, 2012, 9:07 amHi,
Can you give me some tutorial for android with fusioncharts.
Hrishikesh Choudhari
July 19, 2012, 4:10 pmHi Ragav,
We don’t have a sample project which uses the Android WebView with FusionCharts XT. However, we are pretty sure the general idea behind the implementation would be the same.
If you would want to figure out the device orientation using the Android SDK, I suggest you look at – http://developer.android.com/reference/android/content/res/Configuration.html
ragav
July 19, 2012, 3:49 pmHi,
Can you give me some tutorial for android with fusioncharts.
Nandha
July 18, 2012, 12:16 amHi,
Thanks for the great tutorial.
I tried this example, b ut am not able to get this chart. I am getting the “Chart will render here” message on the view.
Please help me to solve this issue.
Thanks,
Nandha
Hrishikesh Choudhari
July 18, 2012, 11:18 amHi Nandha,
Please check the path for the JavaScript files. The div text is displayed when the FusionCharts JavaScript files are inaccessible.
You could also check if the HTML & XML strings are proper by writing them to the Xcode console.
Manuel
July 25, 2012, 1:14 amHi, this tutorial is awesome and you can understand it pretty good… but I am trying to put the graph as a extra view in a view controller… like a small chart in the bottom of the view linked to the variables given by some labels in the view controller, is there anyway you know a tutorial about this? or can you explain this please? I really need your help…
1… resizing the chart to look smaller and in a corner
2… link the bars (in the graph) with some label, so the user can type the numbers
Thanks I really appreciate
Hrishikesh Choudhari
July 25, 2012, 3:18 pmHi Manuel,
Glad you liked the tutorial!
When you want to configure the chart according to some variables, you would need to follow the same technique as we did to create chart data using Twitter API. Simply pick the variables and include them while constructing the XML string.
You could reduce the size of the chart by (a) reducing the dimensions of the UIWebView, or (b) by setting the chart dimensions in pixels. In this tutorial, we’ve specified the chart dimensions in percentages.
Can you please explain what you mean by “link the bars … so the user can type”?
Manuel
July 25, 2012, 7:51 pmI want two bars in the UIWebView… and the user will type a variable in a textfield, then the graph will shows the results… can you please give me a code example of how to do this? how to link the bars with some textfields or labels from a different view controller… I am new with Xcode and can’t find a way to connect this together with the graph…
Hrishikesh Choudhari
July 26, 2012, 11:06 amHi Manuel,
You need to use the values of the text fields when constructing the chart XML.
Look for this line:
[self.chartData appendFormat:@"<set label='%@' value='%@' />", [dateFormatter stringFromDate:[NSDate dateWithTimeIntervalSinceNow:-(i+1)*86400]], [histogramData objectAtIndex:i]];
In this tutorial, we’re providing Twitter data when constructing the XML.
Instead, you will need to pass your variables:
[self.chartData appendFormat:@"<set label='%@' value='%@' />", variable_from_text_field, variable_from_text_field];
This change should get you what you want.
Vamsi
July 31, 2012, 8:59 pmHi,
Thanks for the great tutorial.
I had tried the sample approcah and able to present the graphs on my ipad app.
But one area where I am confused about drill down charts. In the Web sample , I had seen when user clicks a chart it is getting redirected to particular page and the appropriate graphs are getting displayed.
So how to do the same using ios? I mean how to detect the click on a particular bar and render apprpriate graph in ios?
Hrishikesh Choudhari
August 1, 2012, 11:09 amHi,
The functionality that you are asking for is provided by LinkedCharts. Please have a look at the documentation for LinkedCharts.
Thanks.
Gyanendra Kumar
August 27, 2012, 3:59 pmHi,
Is it possible to make line graph through the sample code? If it is then please post some code.
Thanks
Hrishikesh Choudhari
September 10, 2012, 11:10 amHi,
Modifying the sample code to create a line chart would involve changing
Column3D.swf
toLine.swf
in this line:[self.htmlContent appendFormat:@"var chart_object = new FusionCharts('Column3D.swf', 'twitter_data_chart', '%f', '%f', '0', '1');", self.chartWidth, self.chartHeight];
Vanita
August 28, 2012, 5:49 pmHi
Thanks for this great tutorial.
There’s an link below “Fusion Charts” that redirects to Fusion Charts Website. How can that be removed. If it can be resolved using the paid version please let me know the criteria. Thanks in advance.
Hrishikesh Choudhari
September 10, 2012, 11:14 amHi Vanita,
The ‘FusionCharts’ link that you talk about is present in all Evaluation licenses.
This link would not appear in the paid version.
You can visit http://www.fusioncharts.com/buy/ to proceed with the purchase.
Juan Gonzalez
October 15, 2012, 9:28 pmHi again,
do you guys have a newer version of this integration? Maybe something using the native JSON bundled with iOS since version 5?
Thanks and keep the good work!
Leo Joseph
November 7, 2012, 12:24 pmHi, Its an awesome tutorial and visualization. Good that my friend suggested me about this Charts. I used it and my manager got impressed too…. Thanks a lot.
The only problem is how can I get the piechart done?
Leo Joseph
November 7, 2012, 12:25 pmHi, Its an awesome tutorial and visualization. Good that my friend suggested me about this Charts. I used it and my manager got impressed too…. Thanks a lot.
The only problem is how can I get the piechart done?
Thanks again.
Leo Joseph
November 7, 2012, 12:41 pmI got the answer… I just replaced Line with Pie2D.
Ravi
December 10, 2012, 4:02 pmHow did you show Line graph . Please tell me.
Swarnam
December 10, 2012, 6:59 pmHey Ravi,
To render a Single series line chart, modify Column3D.swf to Line .swf using the following code:
[self.htmlContent appendFormat:@”var chart_object = new FusionCharts(‘Line.swf’, ‘twitter_data_chart’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
Sanjukta
November 7, 2012, 1:06 pmHi Leo,
Please confirm if you are willing to create the Pie chart using the above sample code.
In case yes, please try modifying the sample code to create a Pie chart, which would involve changing Column3D.swf to Pie2D.swf / Pie3D.swf, as per your requirement, in this line:
[self.htmlContent appendFormat:@”var chart_object = new FusionCharts(‘Pie2D.swf’, ‘twitter_data_chart’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
Naveen
November 7, 2012, 5:47 pmHi thanks for the tutorial i tried to implement in one of my project using tabbar .my code goes like this ..
organ = [[NSArrayalloc] initWithObjects:@”kidney”,@”liver”,@”brain”,@”heart”,@”health”, nil];
array2 = [[NSArrayalloc] initWithObjects:@”10″,@”20″,@”30″,@”40″,@”50″, nil];
array3 = [[NSArrayalloc] initWithObjects:@”10″,@”10″,@”80″,@”1000″,@”30″, nil];
array4 = [[NSArrayalloc] initWithObjects:@”20″,@”40″,@”60″,@”80″,@”1000″, nil];
array1 = [[NSDictionaryalloc] initWithObjectsAndKeys:@”array2″,@”2″,@”array3″,@”3″,@”array4″,@”4″, nil];
// Done using the connection.
self.currentOrientation = self.interfaceOrientation;
[selfcreateChartData:self.currentOrientation];
}
#pragma mark –
#pragma mark Orientation Support
// Override to allow orientations other than the default portrait orientation.
– (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation {
// Return YES since we support all orientations.
returnYES;
}
// Handle interface rotation.
– (void)willRotateToInterfaceOrientation:(UIInterfaceOrientation)toInterfaceOrientation duration:(NSTimeInterval)duration
{
self.currentOrientation = toInterfaceOrientation;
[selfremoveChart];
[selfcreateChartData:self.currentOrientation];
}
– (void)displayDataError
{
NSMutableString *displayErrorHTML = [NSMutableStringstringWithString:@”<html><head>”];
[displayErrorHTML appendString:@”<title>Chart Error</title>”];
[displayErrorHTML appendString:@”</head><body>”];
[displayErrorHTML appendString:@”<p>Unable to plot chart.<br/>Error receiving data from Twitter.</p>”];
[displayErrorHTML appendString:@”</body></html>”];
[self.webView loadHTMLString:displayErrorHTML baseURL:nil];
}
– (void)createChartData:(UIInterfaceOrientation)interfaceOrientation
{
if (self.twitterDataError) {
[selfdisplayDataError];
} else {
if (interfaceOrientation == UIInterfaceOrientationPortrait || interfaceOrientation == UIInterfaceOrientationPortraitUpsideDown) {
self.chartWidth = 100;
self.chartHeight = 240;
} elseif (interfaceOrientation == UIInterfaceOrientationLandscapeLeft || interfaceOrientation == UIInterfaceOrientationLandscapeRight) {
self.chartWidth = 440;
self.chartHeight = 280;
}
self.chartData = [NSMutableStringstring];
[self.chartDataappendFormat:@”<chart caption=’Your health’ subCaption=’Till now’ showValues=’0′>”];
for (int i = 0; i < [array3 count]; i++) {
[self.chartDataappendFormat:@”<set label=’%@’ value=’%@’ />”, [organobjectAtIndex:i] , [array3objectAtIndex:i]];
}
[self.chartData appendFormat:@”</chart>”];
self.htmlContent = [NSMutableStringstringWithFormat:@”%@”, @”<html><head>”];
[self.htmlContentappendString:@”<script type=’text/javascript’ src=’FusionCharts.js’></script>”];
[self.htmlContentappendString:@”</head><body><div id=’chart_container’>Chart will render here.</div>”];
[self.htmlContentappendString:@”<script type=’text/javascript’>”];
NSLog(@”this scripttype %@”,htmlContent);
[self.htmlContentappendFormat:@”var chart_object = new FusionCharts(‘Pie2D.swf’, ‘twitter_data_chart’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
NSLog(@”this append format %@”,htmlContent);
[self.htmlContentappendFormat:@”chart_object.setXMLData(“%@”);”, self.chartData];
NSLog(@”this append formatXml %@”,htmlContent);
[self.htmlContentappendString:@”chart_object.render(‘chart_container’);”];
NSLog(@”this appendString %@”,htmlContent);
[self.htmlContentappendString:@”</script></body></html>”];
NSLog(@”this append string %@”,htmlContent);
// Draw the actual chart.
[self plotChart];
}
}
– (void)plotChart
{
NSURL *baseURL = [NSURLfileURLWithPath:[NSStringstringWithFormat:@”%@”, [[NSBundlemainBundle] bundlePath]]];
[self.webViewloadHTMLString:self.htmlContentbaseURL:baseURL];
}
everything is working fine but the graph is not loading instead iam getting a text showing Graph will be rendered here . please help me thanks in advance.
Naveen
November 7, 2012, 5:53 pmi have imported all the js files to support it and also jsonkit along with it.
still when i run it, it says graph will berendered here.
and the graph is not shown.
can you tell me why?
Thank you.
Swarnam
November 19, 2012, 10:26 amHi Naveen,
“Chart will render here.” message appears when the FusionCharts JavaScript files are inaccessible.
Please check have you pasted the JavaScript files (FusionCharts.js, FusionCharts.HC.js, FusionCharts.HC.Charts.js and jquery.min.js) in the required folder?
You could also check if the HTML & XML strings are proper by writing them to the Xcode console.
Elakya
February 25, 2014, 3:03 pmHi, im facing the same problem. I have given the absolute path and tested. Same “Chart will load here” only coming.
Swarnam
February 27, 2014, 11:00 amHi Elakya,
As mentioned earlier, the message appears when the FusionCharts JavaScript files are inaccessible.
Also, ensure there are any JavaScript syntax or runtime errors that might have halted the execution of FusionCharts APIs. To debug, you could check if the HTML & XML strings are proper by writing them to the Xcode console.
Ashok Yalamanchili
December 3, 2012, 8:47 amDoes the library plot Pie 3D charts? I have tried changing the line
[self.htmlContentappendFormat:@”var chart_object = new FusionCharts(‘Column3D.swf’, ‘twitter_data_chart’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
to
[self.htmlContentappendFormat:@”var chart_object = new FusionCharts(‘Pie3D.swf’, ‘twitter_data_chart’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
but still the app renders a Pie2D chart. Do I need to change any other parameter for rendering a Pie 3D chart?
Swarnam
December 3, 2012, 2:18 pmHey,
Yes, FusionCharts supports plotting of Pie 3D charts. You do not require to change any other parameters.
To plot a Pie 3D chart, try using the JavaScript files from FusionCharts XT (v3.2.2 – SR3). Prior to this version, Pie 3D chart will render as Pie 2D
Ashok Yalamanchili
December 5, 2012, 9:37 amHey Swarnam,
Thanks for responding. I was able to plot the Pie3D chart bys using the latest JS files from Fusion charts. Do you have any link with the capabilities of the current version of Fusion Charts XT. Because I am trying to plot a hlineargauge chart, but the chart says the “Chart type not supported”
Swarnam
December 5, 2012, 9:40 pmHi Ashok,
To render a HLinearGauge chart, FusionCharts.HC.Widgets.js file from FusionWidgets XT pack has to be present in the same folder as FusionCharts.js.
Vivek
January 14, 2013, 8:23 pmThanks for the good tutorial.
However I have been facing an issue where upon redrawing the chart maybe say on a button click, there is a flicker. Wanted to know if there is any way to remove this.
A possibility could be because the control is completely removed and the white background is shown in the short interval before it redraws again. Is there a way to retain the background of the control and only animate the bars when i wish to redraw the chart?
Swarnam
January 23, 2013, 4:06 pmHi Vivek,
No, it is not possible to redraw only the data plots while updating.We are working on it to obtain a smooth transition and avoid flickering.
Hope this helps.
Vivek
June 4, 2013, 4:28 pmThanks.
Rajmohan
June 26, 2014, 8:35 pmHi How can I remove the white background from chart? The chart is plotting after a gap in webview, its not starting from actual starting point. Can u help me plzz.
Swarnam
June 30, 2014, 5:50 pmHi Raj,
The background color of the chart is fully customizable. Use “bgColor” to set the background color for the chart.
Check if any new line/white space appears before the FusionCharts constructor?
Suresh
April 11, 2013, 6:34 pmHI,
How i can pass these type of data to get chart in IOS.
<?xml version=”1.0″ encoding=”UTF-8″?>
<chart formatNumberScale=”0″ paletteColors=”98ca21,3567b1,f06c29″ showValues=”0″ xAxisName=”shanthi” yAxisName=”Minutes”>
<categories>
<category />
</categories>
<dataset>
<set value=”20″ toolText=”shanthi{br}20/250 Min” />
</dataset>
<dataset>
<set value=”230″ toolText=”shanthi{br}20/250 Min” />
</dataset>
<dataset>
<set />
</dataset>
</chart>
Swarnam
April 15, 2013, 2:58 pmHi Suresh,
Yes, it is possible to get these type of data in iOS. The above XML data format is for Multi Series chart type. So while passing the chart type to the FusionCharts constructor, please specify the Multi Series chart type as per your requirement.
Ref. Code:
[self.htmlContent appendFormat:@“var chart_object = new FusionCharts(‘MSColumn3D.swf’, ‘twitter_data_chart’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
Suresh
April 16, 2013, 11:33 amThanks Swarnam,
Actually i need to pass paletteColors to show the multiple colours in single bar!
That’s should be in Horizontal bar charts! Is it possible to do that in Mobile(IOS) version?
Venkat Nathan
April 12, 2013, 6:51 pmIs it possible to show multiple values within a single bar (base value in green, exceeded value in red etc within a single bar)? We have done this using using the Fusion charts in the browser but not sure if this capability exists now in the mobile version.
Swarnam
April 15, 2013, 3:01 pmHi Venkat,
If it was possible to display multiple values within a single bar in the browser, the same can be achieved in the mobile version. Starting FusionCharts XT we support automatic fallback and the same code can be executed in both browser/mobile version without changing a single line of code.
Sergio Varela
July 13, 2013, 4:23 amHi! I tried compiling your project, but what I receive is a “No data to display” where the graph is suppossed to be, I inserted a flag (actually a NSLog(“Here!”);) right after self.twitterDataError = YES; in order to know if there was a Twitter connection error, but no, is not a Twitter connection error, so I´m suspecting this has to see with the the Twitter API, as this was made with iOS 4.2 in mind and now we are in last iOS 6 version (next release will be 7), any idea what´s going on?? thanx i.a.
Swarnam
July 18, 2013, 9:24 pmHi,
The message “No data to display” occur since the API key for topsy.com been expired.
Can you please regenerate the API key using and append the API key to the below code?
self.twitterQuery = [NSMutableString stringWithFormat:@"%@", @"http://otter.topsy.com/searchhistogram.json?q=html5&slice=86400&period=7"&apikey=SDLXOEYG5E5NQP6HQYLQAAAAAB3K5VL55NIQAAAAAAAFQGYA];
Sergio Varela
July 19, 2013, 11:24 pmThank you Swarnam, it worked great, just a misplaced “, the correct placement is right before the closing ]:
self.twitterQuery = [NSMutableString stringWithFormat:@”%@”, @”http://otter.topsy.com/searchhistogram.json?q=html5&slice=86400&period=7&apikey=SDLXOEYG5E5NQP6HQYLQAAAAAB3K5VL55NIQAAAAAAAFQGYA”];
Ankit
August 21, 2013, 1:28 pmHow can we direct use JSON data with fusion ? I am trying to implement chart in IOS app, it`s working fine with XML data using “setXMLData“, but i tried with “setJSONData” and pass json string in it but i always get Message “Chart will render here.“
Swarnam
September 18, 2013, 1:10 pmHey Ankit,
Can you please check the JSON data for any JavaScript syntax or runtime errors that might have halted the execution of FusionCharts functions?
Abhay Goel
January 9, 2014, 3:02 pmHi,
I am trying to load fusion Chart in my iPhone application. I am getting message in webview “Chart will render here.” but chart is not loading.
The Required JS file is attached in code and i am sending following HTML
Chart will render here.var chart_object = new FusionCharts(‘Charts/AngularGauge.swf’, ‘twitter_data_chart1’, ‘295.000000’, ‘243.000000’, ‘0’, ‘1’);chart_object.setCurrentRenderer(‘javascript’);chart_object.setDataXML(”
“);chart_object.render(‘chart_container’);
Please help me in this issue.
Swarnam
January 9, 2014, 5:24 pmHey,
“Chart will render here.” message appears when the FusionCharts JavaScript files are inaccessible. Please ensure you’ve copied jquery.min.js, FusionCharts.HC.js and FusionCharts.HC.Widgets.js in the same folder as FusionCharts.js.
Also, ensure there are any JavaScript syntax or runtime errors that might have halted the execution of FusionCharts APIs. To debug, you could check if the HTML & XML strings are proper by writing them to the Xcode console.
Hope this helps.
Anoop Thomas
July 30, 2014, 2:42 pmNice article. Thanks
Can we remove FusionCharts watermark at the bottom. We have licensed version of fusion charts V3.2.
Thanks
Anoop Thomas
Swarnam
August 1, 2014, 3:28 pmHi Anoop,
To remove watermark, replace the JavaScript files/SWF Trial files with licensed files.
Clear your browser cache – very important step.
Marx
September 19, 2014, 4:04 pmCan somebody paste here the content of an example JSON file, which could be a replacement of the file from the following link: “http://otter.topsy.com/searchhistogram.json?q=html5&slice=86400&period=7”. The API id expired and the example app cannot display the chart (return a message like “no data to display”).
Sanjukta Mukherjee
September 19, 2014, 5:58 pmHi Marx,
The message “No data to display” occur since the API key for topsy.com been expired.
Can you please regenerate the API key using and append the API key to the below code?
self.twitterQuery = [NSMutableString stringWithFormat:@”%@”, @”http://otter.topsy.com/searchhistogram.json?q=html5&slice=86400&period=7″&apikey=SDLXOEYG5E5NQP6HQYLQAAAAAB3K5VL55NIQAAAAAAAFQGYA”];
Marx
September 23, 2014, 12:57 pmFor those who don’t want to play with API key, here is an example definition of JSON for this app. Just place it somewhere on the web (or local server like MAMP), and modify the self.twitterQuery assignment. Looks pretty cool.
{
“status”: 200,
“request”: {
“url”: “http://api-int:7000/searchhistogram.json?q=html5&slice=86400&period=7”,
“resource”: “searchhistogram”,
“response_type”: “json”,
“parameters”: {
“q”: “html5”,
“slice”: “86400”,
“period”: “7”
}
},
“response”: {
“histogram”: [2839, 3748, 4223, 3530,2444, 1200,4333, 500, 4555],
“period”: 300,
“count_method”: “target”,
“slice”: 86400
}
}
shilpi
September 25, 2014, 11:08 amThanks Marx for sharing this 🙂
Pradeep
October 14, 2014, 12:27 amHi,
Fabulous work by fusioncharts team !
I’m trying to implement fusionchart to my iphone application. I have downloaded the sample code. When i run the application, it says ‘no data to display’ even though i changed the API key. When i executed the “http://otter.topsy.com/searchhistogram.json?q=html5&slice=86400&period=7&apikey=SDLXOEYG5E5NQP6HQYLQAAAAAB3K5VL55NIQAAAAAAAFQGYA” in address bar, it says error: invalid user. What could be the issue ? and also i would like to know how to pass locally stored data to the chart ?
Thanks,
Pradeep
Swarnam
October 14, 2014, 3:59 pmHi Pradeep,
The API key for topsy.com been expired. Can you please regenerate once the API key and append the key to the below code?
Yes, it is possible to pass locally stored data to the chart. It can be done via two ways:
>>Data URL method
>>DataString Method
Ref. Code:
[self.htmlContent appendFormat:@”var chart_object = new FusionCharts(‘MSColumn3D.swf’, ‘strXML’, ‘%f’, ‘%f’, ‘0’, ‘1’);”, self.chartWidth, self.chartHeight];
Instead of retrieving data from Twitter, you will need to generate the data in pre defined format and pass it to the above code.
Hope this helps.
Pradeep
October 16, 2014, 6:48 pmHi Swarnam,
Thanks for your reply. Yes, i have already googled and got some key and it’s working fine. But still i couldn’t figure out how to pass plist data to the chart.
Thanks,
Pradeep
subbu
November 6, 2014, 1:26 pmHi ,
I added one more chart to the above example but it shows “Loading Chart. Please wait”.
Do I need additional js files in my project?
new FusionCharts(‘AngularGauge.swf’, ‘twitter_data_chart6’, ‘%f’, ‘%f’, ‘0’, ‘1’);
data is
bala
February 3, 2015, 7:24 pmHi,
If I use new FusionCharts(‘Pie3D’, ‘twitter_data_chart6′, ‘%f’, ‘%f’, ’0′, ’1′); in iPAD Webview I am not getting Pie chart in 3D format.
Also enablemultislicing is not working in recent version..how to perform that function?
Soarabh
May 28, 2015, 3:57 pmI am getting “Chart type not supported.” error!! HELP!!
Vikas Lalwani
June 1, 2015, 5:55 pmCan you please drop a mail at support[at]fusioncharts.com with more details?