One of the major reasons developers ignore standard technology languages like PHP is the issues faced with the vigor and scalability of the platform. CakePHP is one such robust open-source web framework which is stealing the limelight due to its MVC (model–view–controller) approach, styled along the lines of Ruby on Rails. Even though the framework is based on PHP, it follows a wholly refreshed workflow, making the development process even simpler.
In this post, you’ll learn about CakePHP and how to use it to create data charts and integrate them with FusionCharts Controller.
Table of Contents
Rapid Web Development Enabled by CakePHP
While Agile and Waterfall development approaches are popular amongst developers, Rapid Application Development is gaining traction as it emphasizes the processes involved rather than the planning. As such, it is an ideal approach for developing software that’s driven by user interface requirements. CakePHP is a relatively new framework that provides a reliable base for Rapid Application Development in PHP. This framework follows the MVC (Model-View-Controller) architecture, giving developers a more robust platform for developing apps. CakePHP supports automatic code generation using the console tool Bake, making it more straightforward for developers to develop web apps using Rapid Application Development. Additionally, the framework also supports dynamic scaffolding, allowing developers to define and create a primary application that can create, retrieve, update, and delete objects. Furthermore, it also allows developers to specify how objects are related to each other and develop and break those links. In this tutorial, we will use a MongoDB database to store the data needed to render the chart. Without much ado, let’s get started on using the CakePHP Framework with FusionCharts to create charts.Components to Create Charts in CakePHP
- XAMPP (Server): We will use the XAMPP server for this tutorial. Although, you can use any other server that supports PHP and its frameworks like the MAMP server, the AMPPS server, etc. You can download XAMPP from here; it is available across all major platforms, including Windows, OS X, and Linux.
- CakePHP Framework: CakePHP uses Composer as its package dependency manager. You can install CakePHP using the composer command given below: `php composer.phar create-project –prefer-dist cakephp/app my_app_name` Or if Composer is installed globally: `composer self-update && composer create-project –prefer-dist cakephp/app my_app_name` Read more about installing CakePHP and its packages.
- FusionCharts: You can download the latest version of FusionCharts Suite XT. Alternatively, you can also install it using the npm or bower package managers, using the commands given below:
For npm:
npm install fusioncharts
npm install fusionmaps
For Bower:bower install fusioncharts
bower install fusionmaps
FusionCharts also provides a dedicated PHP wrapper to create charts in PHP. [Download PHP Chart Wrapper]
Step 1. Setting Up the Virtual Host
In this step, we will define a virtual host for our application by adding the code given below in thehttpd-vhost.conf
file of our apache web server.
<VirtualHost *:80> DocumentRoot /path/to/CakePHP framework ServerName cakePHP-tutorial.localhost SetEnv APPLICATION_ENV "development" </VirtualHost>Once we have made the changes in our config file, we will save the file and restart the server. We will now start our CakePHP skeleton application using this [http://cakephp-tutorial.localhost] URL to verify that we are on the right path.
Step 2. Connecting the my_app With the Database
After the successful installation, we noticed that the CakePHP app is still not connected to the database. We need to modify themy_app\config\app.php
file as shown below to establish the connection with the MySQL database.
'Datasources' => [ 'default' => [ 'className' => 'Cake\Database\Connection', 'driver' => 'Cake\Database\Driver\Mysql', 'persistent' => false, 'host' => 'localhost', /** * CakePHP will use the default DB port based on the driver selected * MySQL on MAMP uses port 8889, MAMP users will want to uncomment * the following line and set the port accordingly */ //'port' => 'non_standard_port_number', 'username' => 'db_user_name', 'password' => 'db_user_password', 'database' => 'db_name', 'encoding' => 'utf8', 'timezone' => 'UTC', 'flags' => [], 'cacheMetadata' => true, 'log' => false,
Step 3. Embedding FusionCharts
- PHP wrapper: Download the FusionCharts PHP wrapper and extract. Find the fusioncharts.php file and keep that file inside the folder.
- FusionCharts JavaScript file: We have already downloaded the FusionCharts Suite XT, extract the js file from there and keep that inside a folder named FusionCharts and keep that folder in
my_app/webroot/js/fusioncharts
as shown below – - Open
my_app/src/Template/Layout/default.ctp
file, present in the CakePHP application, and add the js file to that as the format shown below –
Step 4. Setting Up the Fusioncharts Controller
Create the class FusionchartsController below in the filesrc/Controller/FusionchartsController.php
.
<?php namespace App\Controller; use App\Controller\AppController; class FusionchartsController extends AppController { public function index() { } }
Step 5. Creating a View for the FusionchartsController
Start by creating a directory called Fusioncharts undersrc/Template/Fusioncharts
. Now create the file Fusionchart/index.ctp
. This will contain the code required to render the chart.
Let’s create the file index.ctp
inside src/Template/Fusioncharts
, with the following contents:
<?php use Cake\Core\App; //including the FusionCharts PHP wrapper require_once(ROOT .DS. 'vendor' . DS . 'fusioncharts' . DS . 'fusioncharts.php'); use Cake\ORM\TableRegistry; //connecting to the database table $chartData = TableRegistry::get('mscombi2ddata'); //query to fetch the desired data $query = $chartData->find(); //creating the array contains chart attribute $arrData = array( "chart" => array( "animation"=>"0", "caption"=> "Albertsons SuperMart", "subCaption"=> "No. Of Visitors Last Week", "xAxisName"=> "Day", "yAxisName"=> "No. Of Visitors", "showValues"=> "0", "paletteColors"=> "#81BB76", "showHoverEffect"=> "1", "use3DLighting"=> "0", "showaxislines"=> "1", "baseFontSize"=> "13", "theme"=> "fint" ) ); foreach($query as $row) { $value = $row["value1"]; array_push($data, array( "label" => $row["category"], "value" => $row["value1"] ) ); } $arrData["data"]=$data; //encoding the dataset object in json format $jsonEncodedData = json_encode($arrData); // chart object $columnChart = new FusionCharts("column2d", "chart-1", "100%", "300", "chart-container", "json", $jsonEncodedData); // Render the chart $columnChart->render(); ?> // Container to render the chart <div id="chart-container"></div>Now we will render the chart by executing the view page created for the FusionchartsController, via the browser using this URL: http://cakephp-tutorial.localhost/fusioncharts If you’ve followed all the steps, you should have a working chart as shown in the screenshot below:
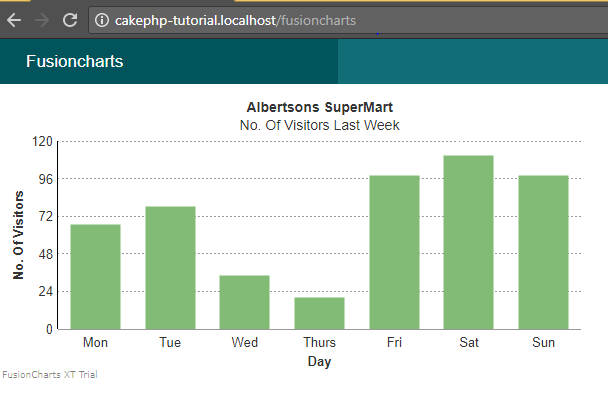
oga samuel
August 21, 2018, 5:20 amInternational charting group