React Native is a JavaScript framework for creating genuine, natively rendered mobile applications for iOS and Android. Based on React, a JavaScript toolkit for creating user interfaces, it focuses on mobile platforms instead of browsers. In other words, using the familiar and beloved JavaScript framework, web developers can now create mobile applications that look and feel native. In addition, React Native makes it simple to simultaneously build for Android and iOS. You can duplicate most of the code you create between platforms. Novice engineers often struggle with how to create pie chart in react native.
For your online and mobile projects, FusionCharts makes it simple to design beautiful dashboards. However, adding engaging and interactive charts is more straightforward, thanks to thorough documentation, cross-browser compatibility, and a standard API. This platform contains all kinds of charts, including simple line charts, column charts, and pie charts, as well as more specialized ones like heatmaps, radar, and stock charts. Read on and learn how to create a React pie chart.
Charts and graphs are simple ways to help companies understand statistical data. The pie chart is a fantastic choice since it’s easy to create and understand when you need to display and quantify simple facts. However, bar graphs or other visualization techniques are preferable to pie charts for more complex uses.
A pie chart is just a circle divided into several portions in two dimensions. The entire graph shows all the data, but individual slices only show a fraction. Suppose you want to show a product line’s efficiency and have two lines that account for 50% of turnover, for example, your pie chart will only have two halves. Some effects are slice pivoting, dragging slices, and three-dimensional charting.
Pie charts are visual, understandable representations. Even the most unskilled audience may find it easy to understand the facts since they are represented visually as a component of a whole. Pie charts enable viewers to analyze data since they can compare two quantitative comparisons. You can alter the data in the pie chart to emphasize points.
React Native graph creation is straightforward using FusionCharts. The complete JavaScript framework for responsive and interactive charts, without a doubt. Include FusionCharts in your web application with only a few lines of code. This platform offers various graph examples. They all have source code, are updated regularly, and are bug-free. Additionally, it provides one-on-one support to help you quickly fix technical issues and information on various charts and dashboards to help you understand all the possibilities. To help you properly visualize your data, FusionCharts also offers a range of graphs.
Click here to start using FusionCharts now that you know how to create pie charts in React Native.
Table of Contents
Are React and React Native the same?
In simple words, no. React and React Native are two different libraries. React is an open-source JS package for creating user interfaces for web applications. It is a collection of reusable parts created to build the frameworks for the programs. While simply requiring JavaScript, React Native is used to create sophisticated mobile user interfaces using declarative components. It helps developers build native mobile apps using reusable parts. Both React and React Native dominate the market and are utilized extensively in online and mobile apps. React, often known as ReactJS, is a free JavaScript package for creating single-page web applications. It is one of the most used libraries for creating online and mobile application UI (front end). You can create quick, flexible, and user-friendly mobile apps with React. They can automate the design process. ReactJS developers may create anything they want without following rigid guidelines. Using libraries or plugins with existing code is simple for ReactJS developers. They may also create quick, scalable, and straightforward web apps thanks to it. React’s introduction to front-end programming brings back the sorely missed server-side logic. Facebook created React Native, an open-source JavaScript-based framework, to satisfy its expanding demand for mobile. With the hybrid mobile app framework React Native, you can create mobile apps from a single code base. With the help of this JavaScript framework, you can create mobile applications that run seamlessly on iOS and Android. In addition, you can develop cross-platform applications with React Native for Windows, Android, and iOS. So another framework for creating cross-platform applications is now available. React Native uses the fundamental abstraction of ReactJS. However, only the library’s parts are unique. ReactJS and React Native differ significantly, although sharing a fundamental essence similar to that of siblings. Both technologies, though, are masters in their fields. The cost of developing an app with React Native also depends on the functional needs.Pie charts: what are they, and what are the benefits?
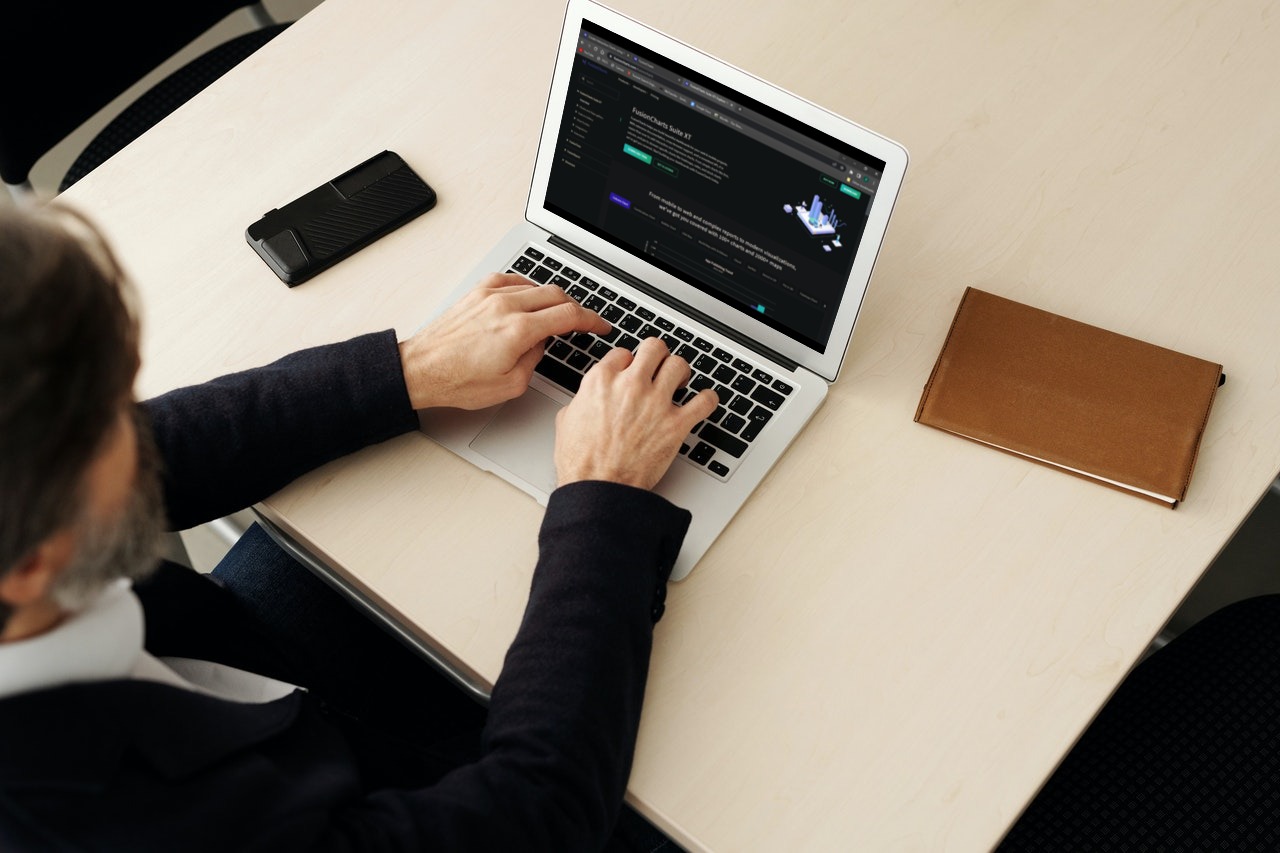
How Can a Pie Chart Be Created in React Native?
Pie charts come in a variety of forms. Click here to view them. We’ll demonstrate how to use FusionCharts to create a few charts.A 2D Pie Chart: How to Create One?
A circle is split into segments in a 2D pie chart. Each segment denotes a proportion of the overall value in the dataset. The graph is a great way to show how many components make up the whole. The dependent variable’s magnitude is inversely related to the arc length on the graph’s circumference. Radial lines join the arcs to the circle’s center and divide the pie into slices. It represents the market share of a brand or product category as a proportion of total sales in a specific industry. Here is an example of a 2D pie chart made with React Native.import React, { Component } from "react"; import { AppRegistry, StyleSheet, Text, View, Platform } from "react-native"; import FusionCharts from "react-native-fusioncharts"; const dataSource = { chart: { caption: "Market Share of Web Servers", plottooltext: "<b>$percentValue</b> of web servers run on $label servers", showlegend: "1", showpercentvalues: "1", legendposition: "bottom", usedataplotcolorforlabels: "1", theme: "fusion" }, data: [ { label: "Apache", value: "32647479" }, { label: "Microsoft", value: "22100932" }, { label: "Zeus", value: "14376" }, { label: "Other", value: "18674221" } ] }; export default class App extends Component { constructor(props) { super(props); this.state = dataSource; this.libraryPath = Platform.select({ // Specify fusioncharts.html file location ios: require("./assets/fusioncharts.html"), android: { uri: "file:///android_asset/fusioncharts.html" } }); } render() { return ( <View style={styles.container}> <Text style={styles.heading}> FusionCharts Integration with React Native </Text> <View style={styles.chartContainer}> <FusionCharts type={this.state.type} width={this.state.width} height={this.state.height} dataFormat={this.state.dataFormat} dataSource={this.state.dataSource} libraryPath={this.libraryPath} // set the libraryPath property /> </View> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, padding: 10 }, heading: { fontSize: 20, textAlign: "center", marginBottom: 10 }, chartContainer: { height: 200 } }); // skip this line if using Create React Native App AppRegistry.registerComponent("ReactNativeFusionCharts", () => App);
A 3D Pie Chart: How to Create One?
A circle divided into sectors, each representing a percentage of the total values in a dataset, makes up the pie 3D graphic. By adding depth, the pie 3D chart elevates the simple pie chart’s visual appeal. The graph is excellent for showing how each constituent’s percentage fits into the overall picture. The radius of the arc on the graph’s circumference is inversely proportional to the size of the dependent variable. The circle’s center is reached by connecting the arcs with radial lines, which cut the pie into slices. It displays the percentage split or contribution of several factors, such as the breakdown of sales by product category or the market share of different brands in a specific industry. Here is an example of a 3D pie chart made with React Native.import React, { Component } from "react"; import { AppRegistry, StyleSheet, Text, View, Platform } from "react-native"; import FusionCharts from "react-native-fusioncharts"; const dataSource = { chart: { caption: "Recommended Portfolio Split", subcaption: "For a net-worth of $1M", showvalues: "1", showpercentintooltip: "0", numberprefix: "$", enablemultislicing: "1", theme: "fusion" }, data: [ { label: "Equity", value: "300000" }, { label: "Debt", value: "230000" }, { label: "Bullion", value: "180000" }, { label: "Real-estate", value: "270000" }, { label: "Insurance", value: "20000" } ] }; export default class App extends Component { constructor(props) { super(props); this.state = dataSource; this.libraryPath = Platform.select({ // Specify fusioncharts.html file location ios: require("./assets/fusioncharts.html"), android: { uri: "file:///android_asset/fusioncharts.html" } }); } render() { return ( <View style={styles.container}> <Text style={styles.heading}> FusionCharts Integration with React Native </Text> <View style={styles.chartContainer}> <FusionCharts type={this.state.type} width={this.state.width} height={this.state.height} dataFormat={this.state.dataFormat} dataSource={this.state.dataSource} libraryPath={this.libraryPath} // set the libraryPath property /> </View> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, padding: 10 }, heading: { fontSize: 20, textAlign: "center", marginBottom: 10 }, chartContainer: { height: 200 } }); // skip this line if using Create React Native App AppRegistry.registerComponent("ReactNativeFusionCharts", () => App);
Why use FusionCharts to make pie charts in React Native?
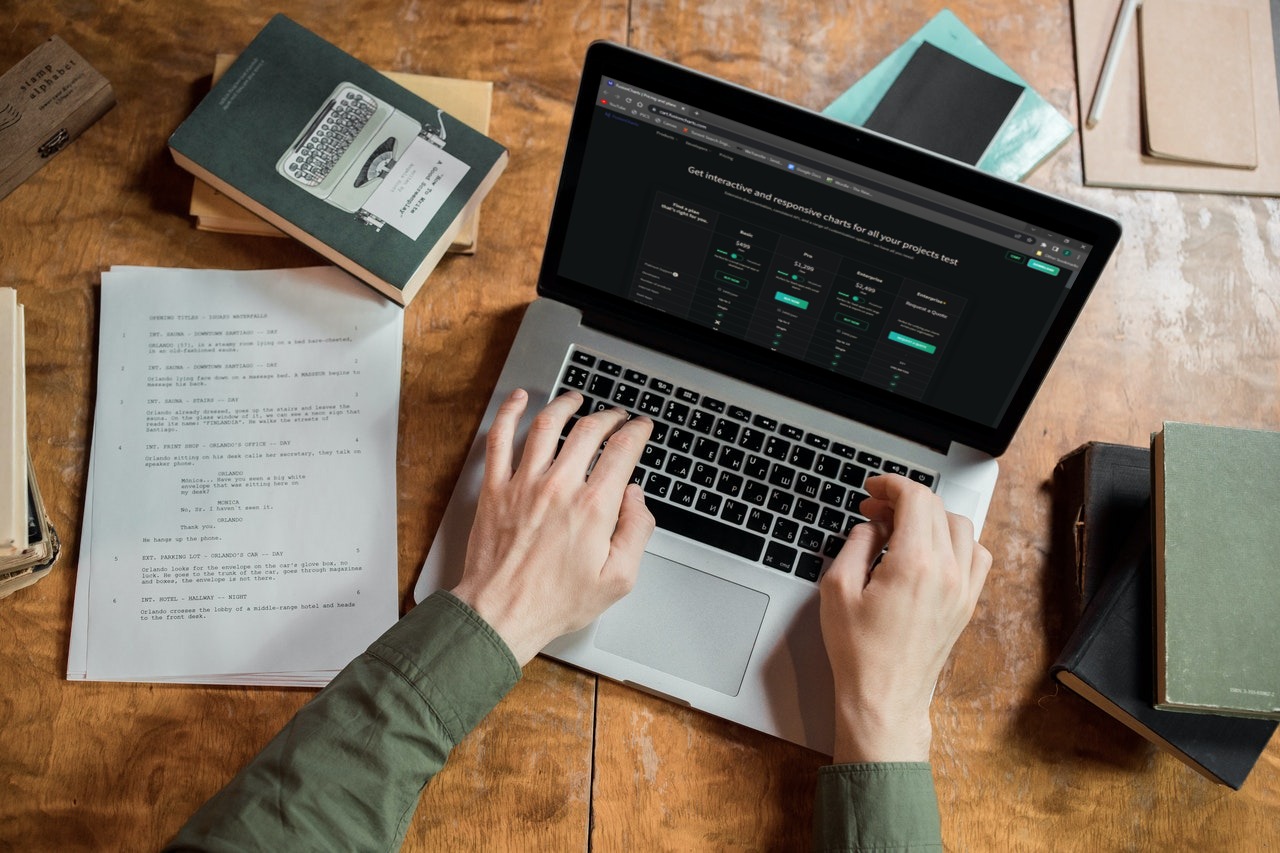