This the second part of the FusionCharts XT with PHP series. We will create interactive JavaScript charts using data from a MySQL database with FusionCharts XT. We will make use of the PHP library that is bundled with the FusionCharts Suite.
To recap from the first article in this series, FusionCharts XT is essentially a JavaScript charting library, which renders highly interactive charts in the browser. It takes data in either XML or JSON. To plot a chart using PHP, you need to include the HTML and JavaScript code to embed a chart object and then provide the requisite parameters. To make things simpler for you, we have put all this functionality in a PHP script named
FusionCharts.php
. This script is bundled in FusionCharts XT Download Package > Code > PHP > Includes > FusionCharts.php
. So, whenever you need to work with FusionCharts XT in PHP, just include this file in your page and you’re good to go.
Table of Contents
FusionCharts XT in PHP Series
- Part 1 – JavaScript charts in PHP using FusionCharts XT
- Part 2 – JavaScript charts in PHP and MySQL using FusionCharts XT
- Part 3 – Drill-down JavaScript charts in PHP and MySQL
- Part 4 – JavaScript LinkedCharts in PHP and MySQL
- Part 5 – Using Debugging Tools for JavaScript Charts
What we are going to visualize
We will make use of the sample World database provided by MySQL and plot the Top 10 Most Populous Countries.What we will need
- PHP running on any server
- MySQL
- The latest version of FusionCharts XT
- The sample World database provided by MySQL. Read more to understand the structure of this database and how to set it up.
- Within the root directory of your web server, create a folder named
FusionCharts_XT_with_PHP
. This will be our demo folder. - Copy the entire
Charts
folder from the FusionCharts XT Download Package and paste it within our demo folder. This completes the installation of FusionCharts XT in our web application. - Copy the
Includes
folder fromFusionCharts XT Download Package > Code > PHP > Includes
to our demo folder.
Connecting to the MySQL database
In theIncludes
folder, we provide you with DBConn.php
, which you can use to connect with your database. You may use this file to authenticate with your database if you aren’t using any other method. You would have to provide the relevant values to connect to your database. For instance, on our demo computer, we have the following values:
// These four parameters must be changed dependent on your MySQL settings $hostdb = 'localhost'; // MySQl host $userdb = 'root'; // MySQL username $passdb = ''; // MySQL password $namedb = $dbName ? $dbName : 'world'; // MySQL database nameThere are two ways using which you can provide data to the PHP library – Data URL and Data String. Let us use both of them in detail.
Creating the chart using the Data URL method
In the Data URL method, you instruct FusionCharts to load XML or JSON data from a URL. This URL could refer to a static XML file that is already present on the server, or it could refer to a virtual data provider e.g.,/path_to/DataProvider.php
, which executes queries the database, builds the XML data as string and finally outputs this XML string to the output stream with content type set to [cciel lang=’html’]text/xml, but without any HTML tags. We then need to create a second file, which would receive this XML string, pass it on to FusionCharts.php
, and then render the chart.
Let us create the first file named FusionChartsXT_with_PHP_and_MySQL_using_DataURL_DataProvider.php
. Write the following code in this file:
< ?php // Set the content type in the header to text/xml. header('Content-type: text/xml'); // Include DBConn.php include('Includes/DBConn.php'); // Use the connectToDB() function provided in DBConn.php, and establish the connection between PHP and the World database in our MySQL setup. $link = connectToDB(); // Form the SQL query which will return the Top 10 Most Populous Countries. $strQuery = 'SELECT Name AS Country, Population FROM country ORDER BY Population DESC LIMIT 10'; // Execute the query, or else return the error message. $result = mysql_query($strQuery) or die(mysql_error()); // If we get a valid response - if ($result) { // Create the chart's XML string. We can add attributes here to customize our chart. $strXML = ""; while($ors = mysql_fetch_array($result)) { // Append the names of the countries and their respective populations to the chart's XML string. $strXML .= ""; } } // Close the chart's XML string. $strXML .= ""; // Return the valid XML string. echo $strXML; ?>Save this file and navigate to it using your web browser. You should see the following XML displayed: We now need to pass this XML data to
FusionCharts.php
, which will generate the required HTML and JavaScript code to display our chart. Let us create a PHP file FusionChartsXT_with_PHP_and_MySQL_using_DataURL.php
. In this file, write the following code:
< ?php // It would generate the HTML and JavaScript code required to render the chart. include('Includes/FusionCharts.php'); ?> < !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
< ?php // Set the rendering mode to JavaScript FC_SetRenderer(‘javascript’); // Call the renderChart method, which would return the HTML and JavaScript required to generate the chart echo renderChart(‘FusionCharts/Column2D.swf’, // Path to chart type ‘FusionChartsXT_with_PHP_and_MySQL_using_DataURL.php’, // Path to the data provider ”, // Empty string when using Data URL Method ‘top10_most_populous_countries’, // Unique chart ID ‘660’, ‘400’, // Width and height in pixels false, // Disable debug mode true // Enable ‘Register with JavaScript’ (Recommended) ); ?>
Save this file and navigate to it using your web browser. This is the JavaScript chart that you should get to see:
Creating the chart using Data String method
In the Data String method, the XML or JSON data is embedded within a single web page, along with the chart’s HTML and JavaScript code. This method doesn’t require a static data file or a virtual data provider. Moreover, once the chart has finished loading, the data is present locally within the page. Create a blank PHP file namedFusionChartsXT_with_PHP_and_MySQL_using_DataString.php
. Write the following code in this file:
< ?php // Include the DBConn.php and FusionCharts.php files, so that we can access their variables and functions. include('Includes/DBConn.php'); include('Includes/FusionCharts.php'); // Use the connectToDB() function provided in DBConn.php, and establish the connection between PHP and the World database in our MySQL setup. $link = connectToDB(); // Form the SQL query which will return the Top 10 Most Populous Countries. $strQuery = 'SELECT Name AS Country, Population FROM country ORDER BY Population DESC LIMIT 10'; // Execute the query, or else return the error message. $result = mysql_query($strQuery) or die(mysql_error()); // If we get a valid response - if ($result) { // Create the chart's XML string. We can add attributes here to customize our chart. $strXML = ""; while($ors = mysql_fetch_array($result)) { // Append the names of the countries and their respective populations to the chart's XML string. $strXML .= ""; } } // Close the chart's XML string. $strXML .= ""; ?> < !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
< ?php // Set the rendering mode to JavaScript FC_SetRenderer(‘javascript’); // Call the renderChart method, which would return the HTML and JavaScript required to generate the chart echo renderChart(‘FusionCharts/Column2D.swf’, // Path to chart type ”, // Empty string when using Data String method $strXML,// Variable which has the chart data ‘top10_most_populous_countries’, // Unique chart ID ‘660’, ‘400’, // Width and height in pixels false, // Disable debug mode true // Enable ‘Register with JavaScript’ (Recommended) ); ?>
Save this file and navigate to it using your web browser. This is the JavaScript chart that you should get to see:
Download source files for these samples
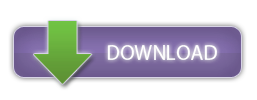
oscar
April 16, 2012, 1:44 pmHie after the whole process, the chart is not displayed but only the space holder “chart” is shown, please help
Hrishikesh Choudhari
April 16, 2012, 2:41 pmHi Oscar,
Check whether the JavaScript files are properly included in the page; make sure their path is correct.
This issue occurs when the FusionCharts JavaScript file is not loaded in the page.
Let us know if this solves your problem..
NTAKIRUTIMANA
May 29, 2012, 8:24 pmthanks
fabien
June 25, 2012, 6:15 pmhi,
In echo renderChart(………) there is FusionChartsXT_with_PHP_and_MySQL_using_DataURL.php and i think it better be
FusionChartsXT_with_PHP_and_MySQL_using_DataURL_DataProvider.php
Thanks for you work !!
Rosenbaum
November 11, 2012, 8:14 amThanks!!!
ketan p
November 22, 2014, 1:59 pmwhat is the actual contents of FusionCharts.php
Ferrer
August 12, 2015, 9:42 pmHi, I have the same question, what is the contents of FusionCharts.php?? And how do I include the FusionCharts.php to a php file??
videogames-info.mex.tl
June 22, 2017, 3:13 pmI blog often and I truly thank you for your content.
This article has truly peaked my interest. I’m going to take a note of
your blog and keep checking for new details about once per week.
I subscribed to your Feed too.
Nursing Test Bank
July 24, 2017, 2:50 amThanks to my father who stated to me regarding this weblog,
this blog is genuinely awesome.
temporary office
April 14, 2018, 9:51 pmHi, I think your site might be having browser compatibility issues.
When I look at your website in Chrome, it looks fine but when opening in Internet Explorer, it
has some overlapping. I just wanted to give you
a quick heads up! Other then that, very good blog!
nap ho ga thanh an
September 4, 2018, 6:43 amFantastic blog! Do you have any suggestions for aspiring writers?
I’m hoping to start my own blog soon but I’m a little lost on everything.
Would you advise starting with a free platform like WordPress or go for a paid option? There are so many options
out there that I’m totally confused .. Any suggestions?
Thank you!
Bandar Ceme Terpercaya
September 24, 2018, 9:55 amYes! Finally something about Judi Poker.
Situs Dominoqq Online Terbaik
December 6, 2018, 5:11 pmHello everyone, it’s my first go to see at this site, and piece of wreiting iss
really fruitful in support of me, kewp uup posting these types of articles oor reviews.