Finding the best data structures or methods to employ in your code can sometimes take time and effort. However, you can save a lot of time, improve the readability of your code, and boost its performance by simplifying it. Using a JS map with different JavaScript map methods is one of the best methods of streamlining your code.
Until ES6, objects in a map were usually used for mapping keys with any value. Using objects for maps can cause side impacts. The ES6 collection-type Map addresses this problem. So in this article, we will understand all these concepts better by diving deep into Map Methods in JavaScript, and we’ll even introduce you to a platform that makes working on JS map methods easy. So let’s get started!
Any data type can be used as a key or value in a JavaScript map, which also retains the order in which its elements appear. A new array can be created by continuously iterating through each element in an array. In other words, you can modify the members of an array and then obtain a new array depending on the function’s output. Because of this, Maps are helpful for quick searches and data lookups.
A JS map can be instantiated Just like any other JS class. However, we must provide some parameters because it will be an empty Map, the same as an empty object. So, if you want to fill the Map with default values, you must supply it with an iterable value where the first value in the inner array is the key and the second value is the value for the key-value pair.
You can also use maps to conduct an action on each item in a collection and compile the results into a new array. The “for loop” or “nesting” constructs in JavaScript can do the same operation; however, we can write more legible functions by using a map.
The new Map() syntax allows for the creation and initialization of a map. You can decide to give it a value at a later time. A new map object can also be created by giving an array to a new Map(). A new map object with a key and associated value will be created.
What happens if we have a sizable codebase and want to dynamically add entries that are not known in advance, even though we can manually add them to the Map? This implies that the Map will require frequent updating. This could be a challenging task. You may need a better method of adding values to the map after creating it. Calling the set method and passing the desired key and value is all required. You can expand your program by adding new entries (key-value pairs).
Values can be assigned and fetched with equal ease. Simply calling the get method with the required key will do. If a key exists, it will either return the map values for that key or be undefined if it cannot be found.
There are times when all you want to do is see if a Map has a particular value saved. You can do this by passing the key you want to check for to the has method.
The final CRUD operation you want to perform is removal, which is also very easy. Simply call delete while passing the key you want to remove.
Note: The key of the entry you want to delete is the only parameter that the .delete() method accepts.
Iteration is the final step, and out of all the methods available, the forEach method is the most frequently used to iterate across a Map. This method calls a callback with a value and key parameters, just like the array forEach function does.
You can use the table below to quickly review the primary characteristics of JS maps (some of which we have already covered in detail above) and comprehend their primary application.
Keys and values are inherent properties of map objects. The Collection Maps contain only one key for each map. Similarly to this, keys on maps are only visible once. Every iterator object returning a Map object returns the same [key value] 2-part array. The order of iterations corresponds to the order in which the key-value pairs were initially entered by setting into the map. This article has covered all the key map-related coding techniques to help you write JS code more effectively.
It’s also important to note that FusionCharts is a platform that will ease Creating Map With Javascript. For example, for displaying business information like revenue by region, employment levels by state, and office locations, FusionMaps XT has over 2000+ geographical maps, including all nations, US states, and European regions, aiding you in Creating Custom Maps for your application.
So, if you want to create stunning JavaScript maps in a few minutes, click here and sign up at FusionCharts!
Table of Contents
What is a Map in JavaScript?
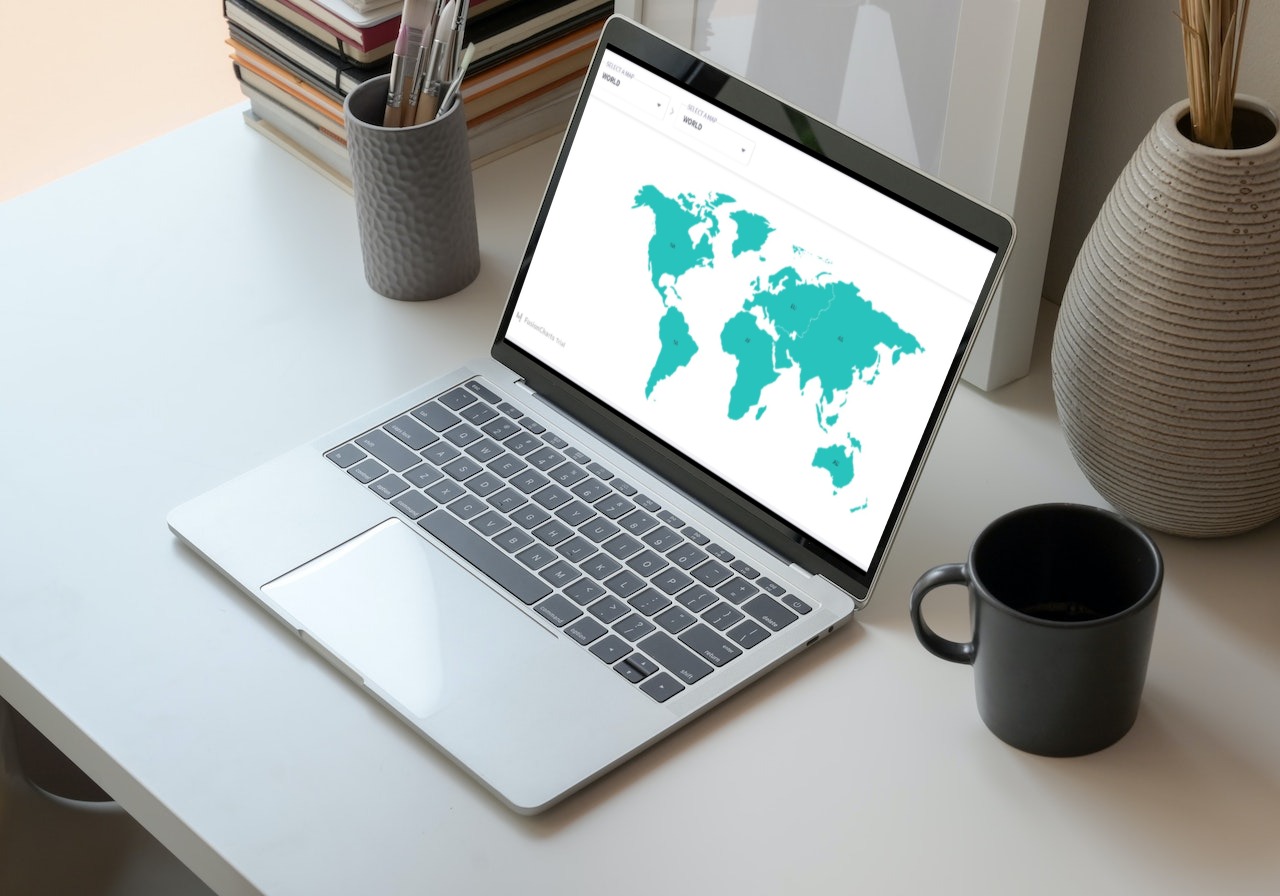
How to Build a Map in JavaScript?
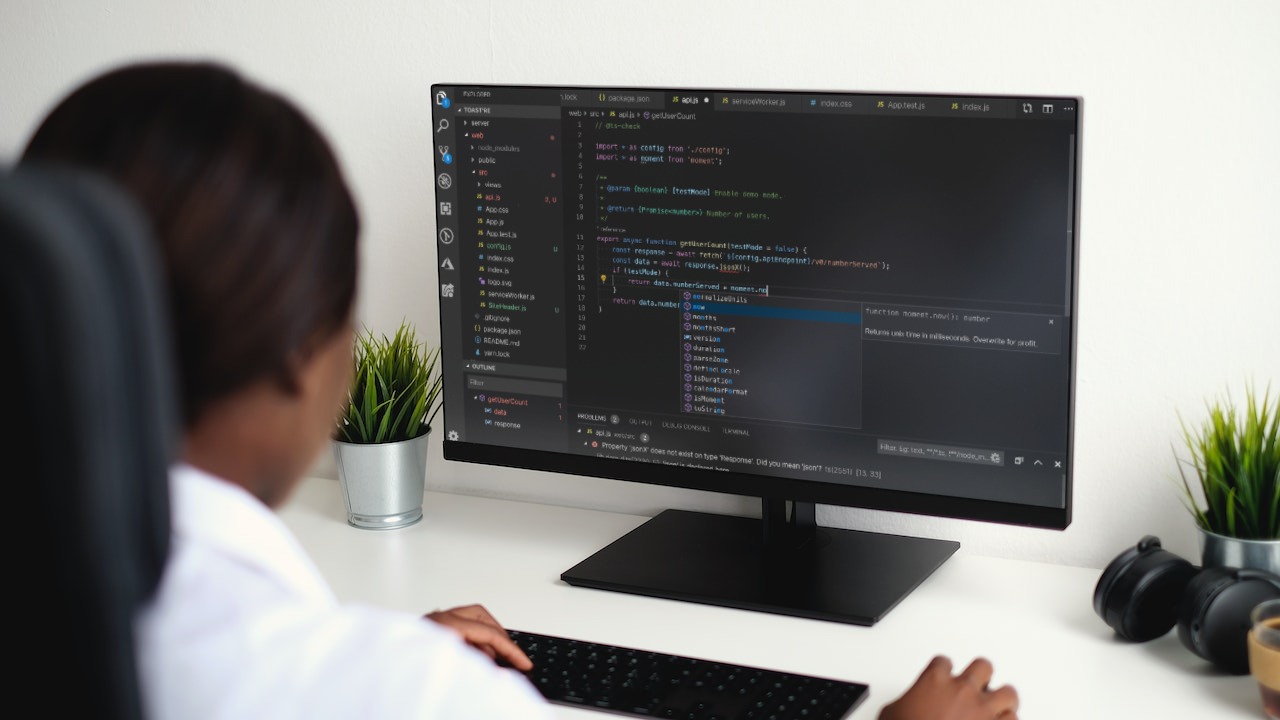
const emptyMap = new Map() const map = new Map([ ['key', 'value'] ])
How to Assign Values to a JS Map?
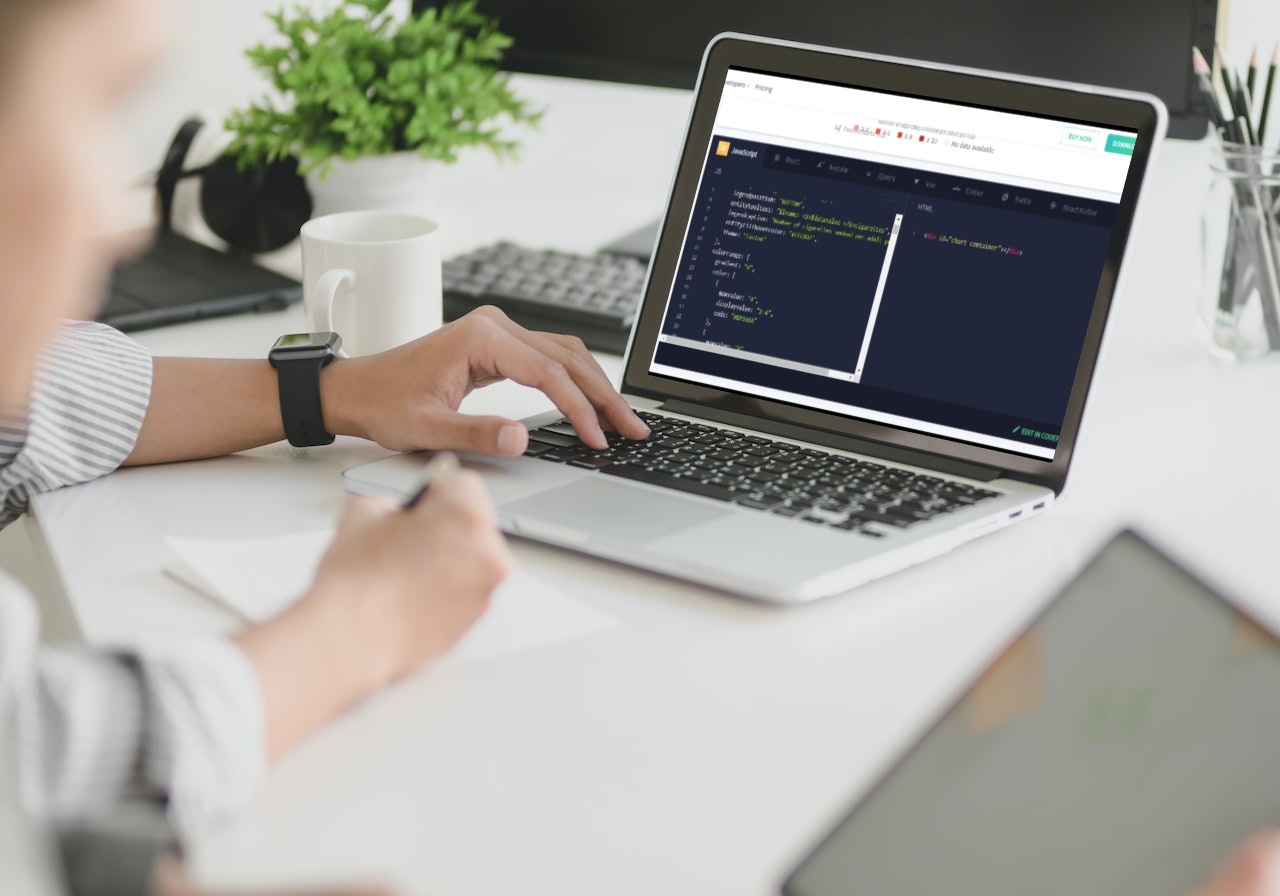
const map = new Map() map.set('key', 'value') map.set(true, 'boolean') // "key" => "value" // true => "boolean"
How Easy is Fetching Values from a JS Map?
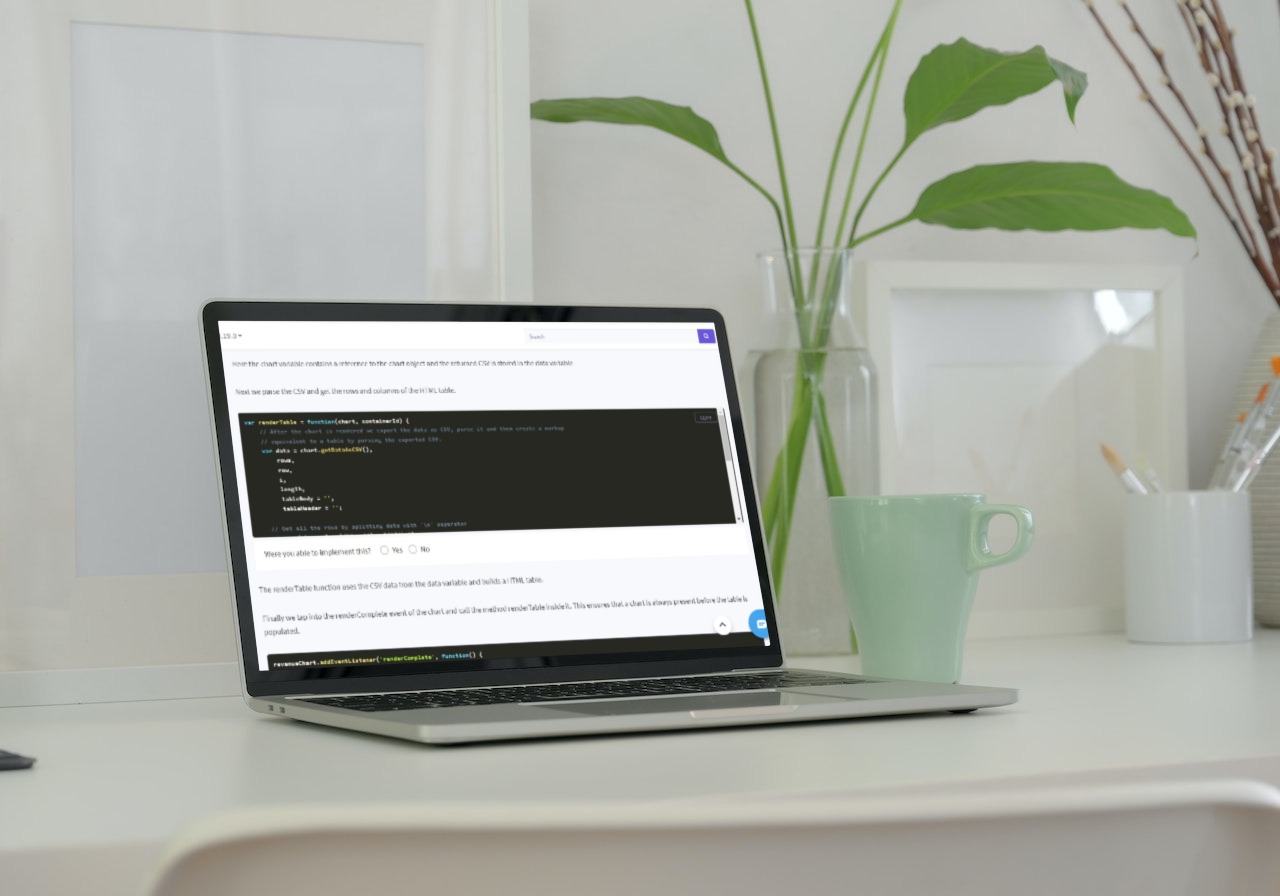
const map = new Map() map.set('key', 'value') map.set(true, 'boolean') map.get('key') // "value" map.get(true) // "boolean" map.get('wrong-key') // undefined
Can You Check Values in a Map?
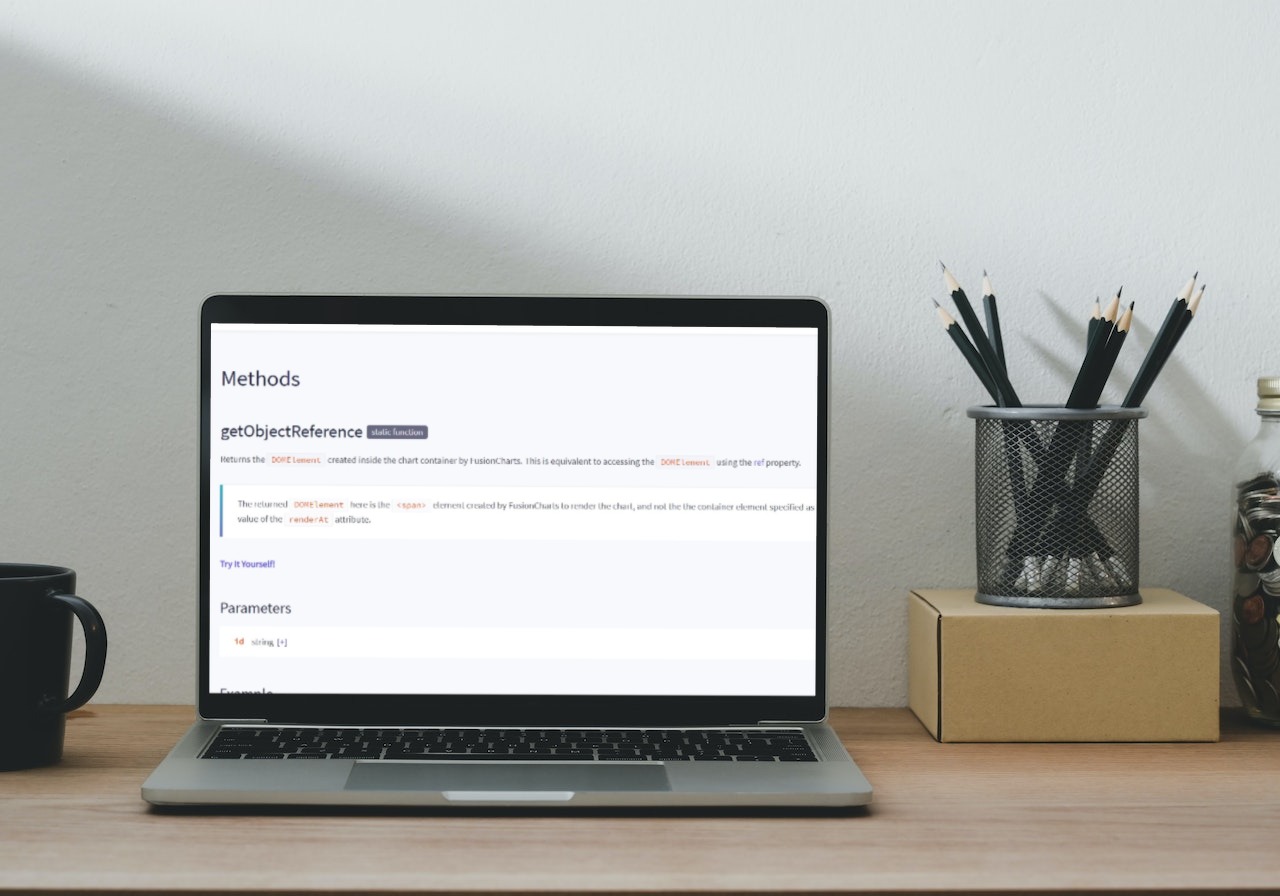
const map = new Map() map.set(1, 'number') map.has(1) // true map.has('1') // false map.has('wrong-key') // false
Can You Even Remove Values from a Map?
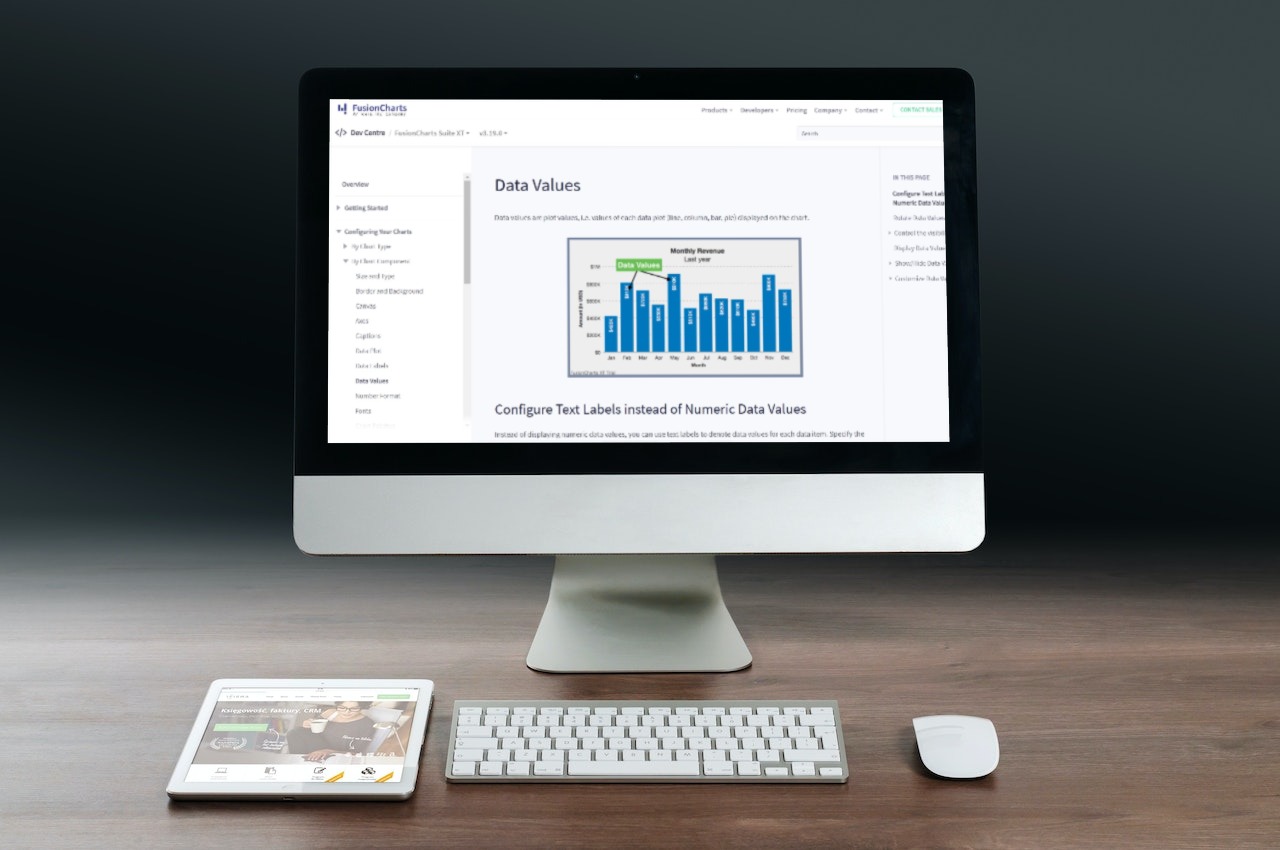
const map = new Map() map.set(1, 'number') map.set('a', 'b') map.delete(1) map.has(1) // falseUsing the delete method can be a laborious process if we want to remove all map elements from the Map, not just one particular entry. The .delete() method allows us to delete each entry one at a time, but doing so will be complex and inefficient. Because of this, you should use the .clear() method to effectively and quickly remove all the entries. Like the .delete() method, the .clear() method deletes entries from the Map. It eliminates all the elements in the Map object rather than just a particular entry.
How Easy is Iteration of Maps in JavaScript?
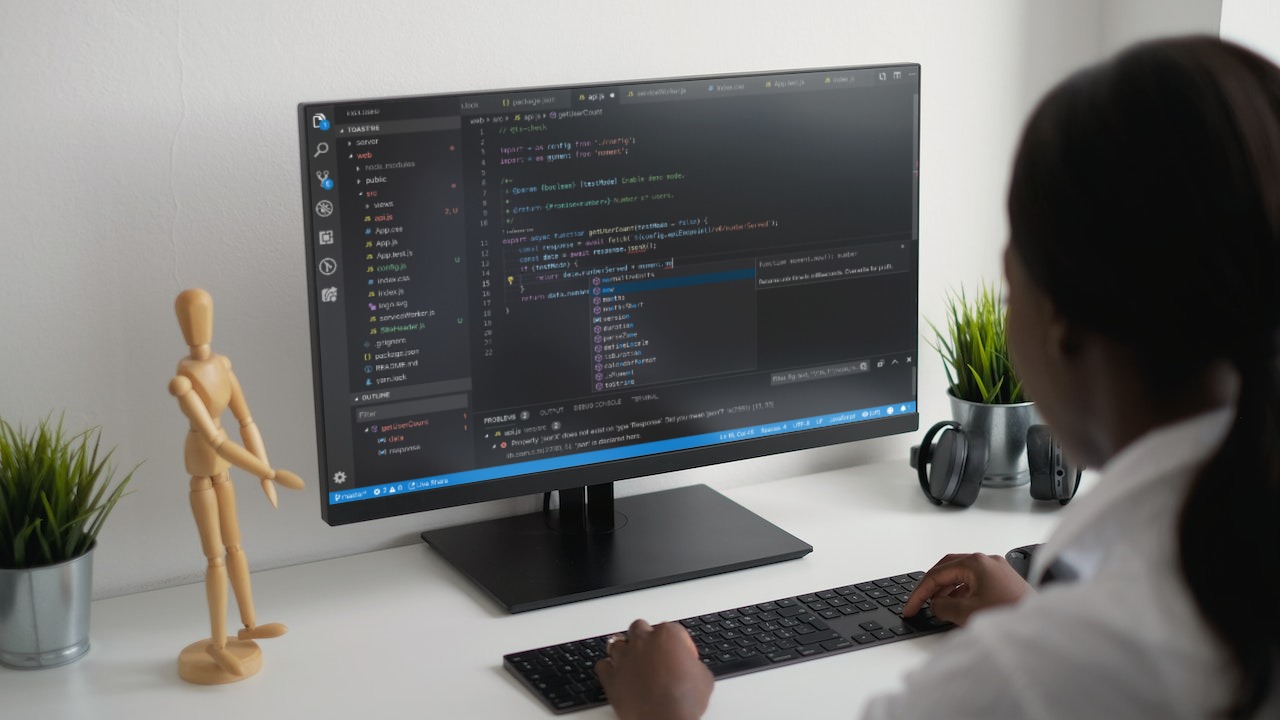
const map = new Map() map.set(1, 'number') map.set('a', 'b') map.forEach((value, key) => { console.log(`${key} => ${value}`) }) // 1 => number // a => bTo iterate through the list of items in the Maps, the forEach() method is used. Again, consider the scenario where we want to print the entries from a Map. This time, however, we want to connect the keys and values using a function rather than the usual arrows pattern.
What are Various Map Properties?
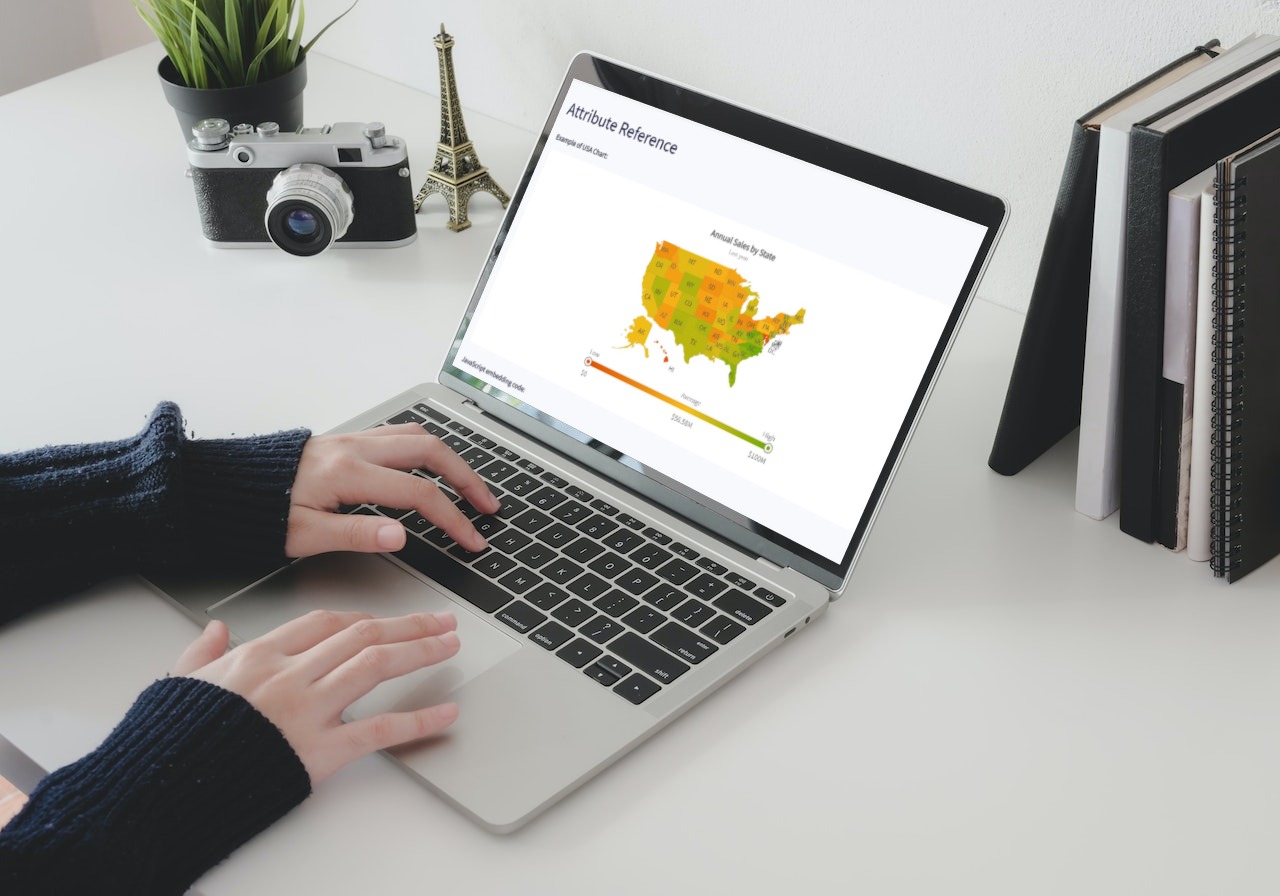
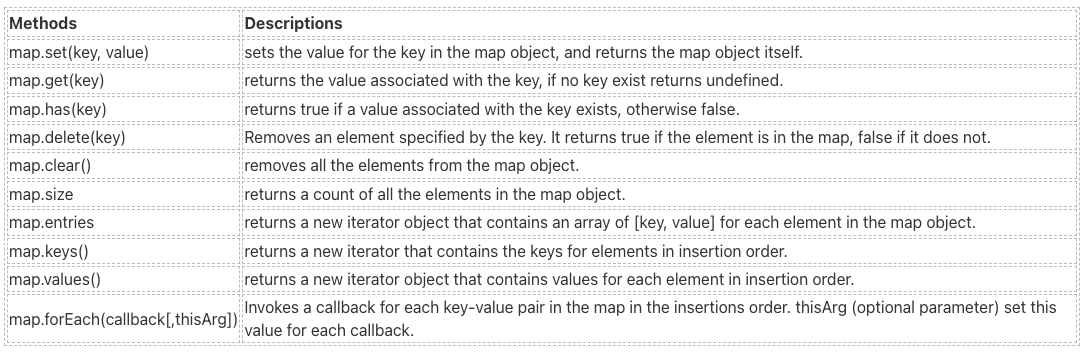
How are Maps Different from Objects in JavaScript?
A major benefit of a map is that it will preserve the order of entries while maintaining the insertion order (There is no guarantee of order for objects). Furthermore, there are no unexpected default keys because a Map only contains the information you explicitly enter. It’s important to remember that because an Object has a prototype, it contains default map keys that might conflict with your keys. Key values in a Map can be more flexible because they can be any value, including functions, objects, and primitives. However, a key can only be a string or symbol in an object. Map’s flexibility with more data types could be useful depending on how you store your data. Although keys in maps are unique, when an object is used as a key, the map will use the object’s reference to check for equality. Remember that two objects in a JavaScript map will not be regarded as equal if they share the same value(s) but not the same reference.What have You Learned about JS Map Methods?
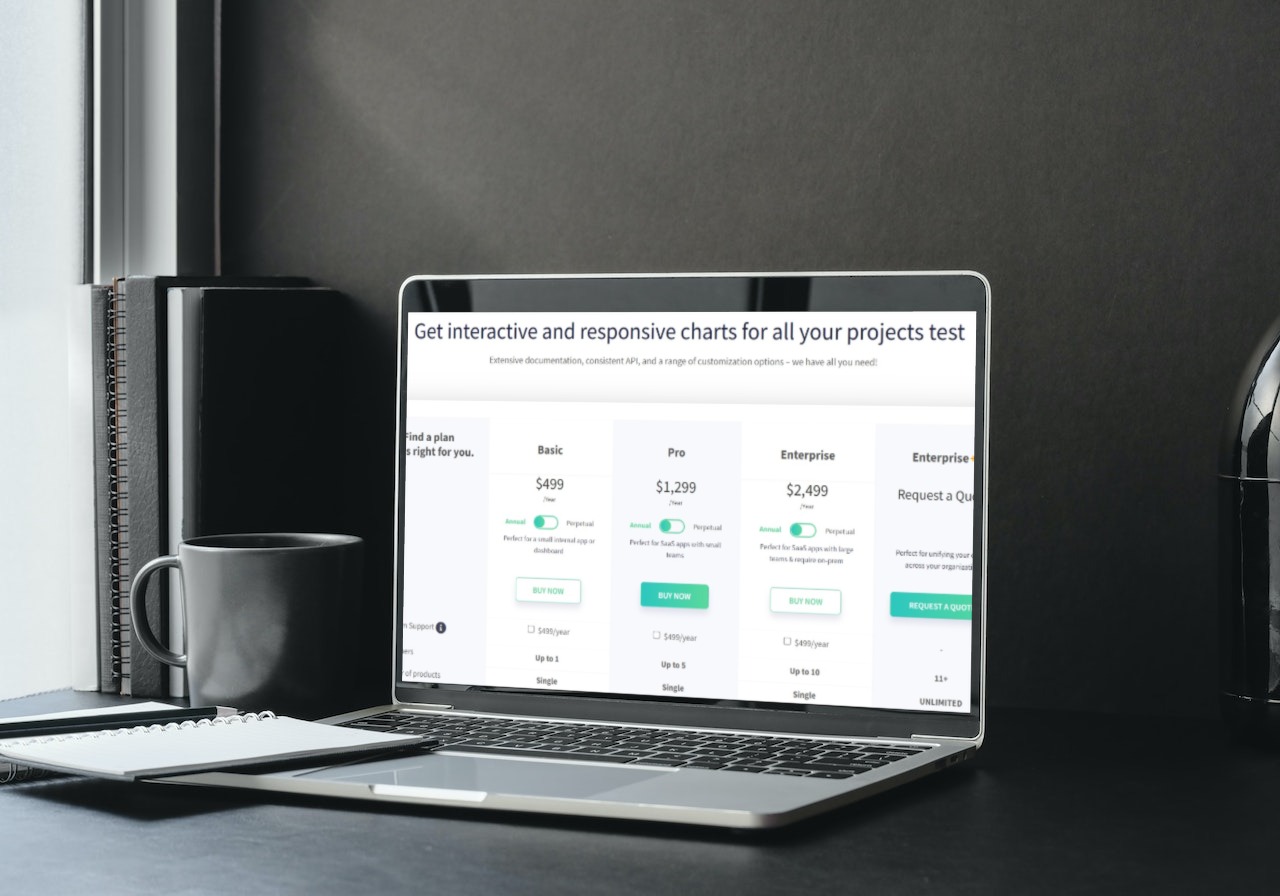
What are Some FAQs Regarding This Topic?
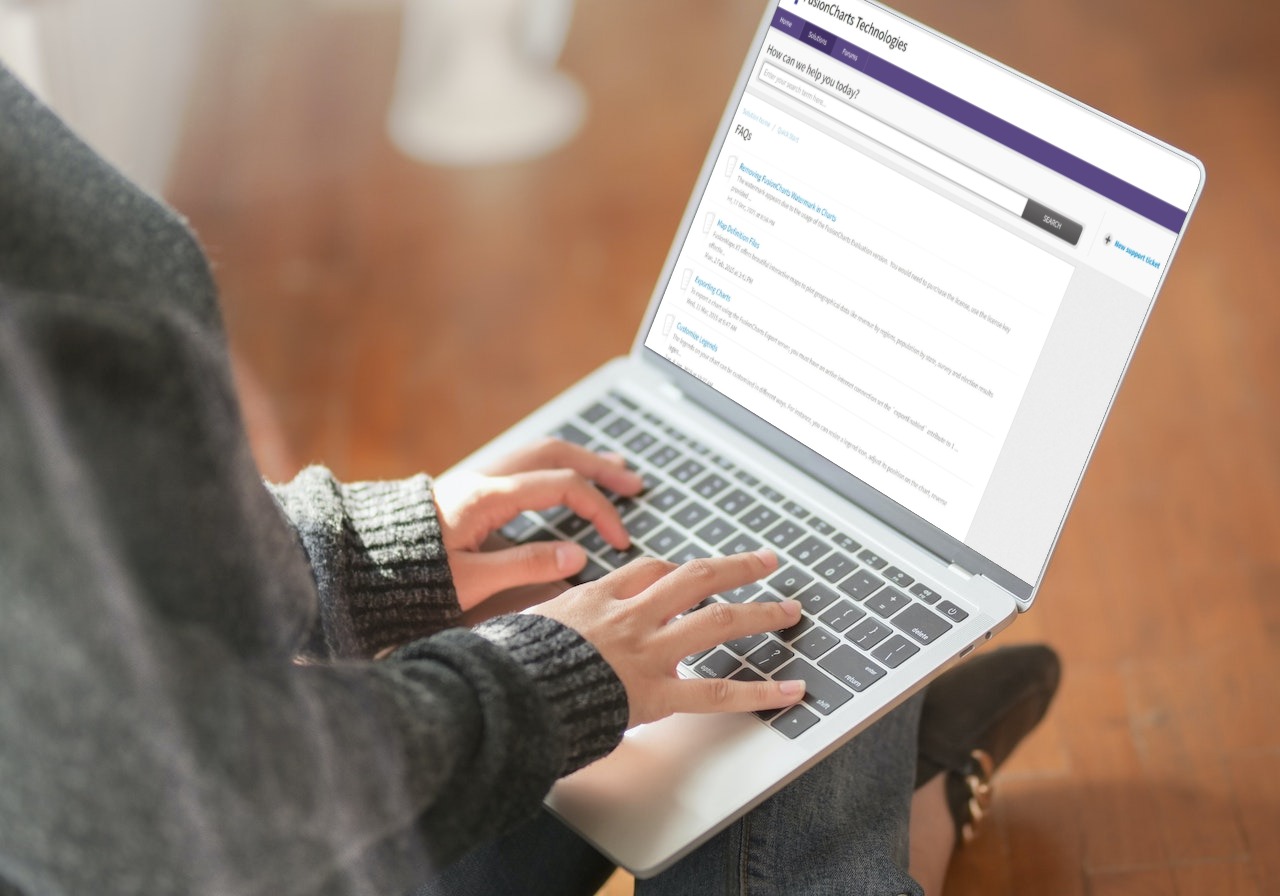